STM32有ds18b20的驱动代码的固件库吗
时间: 2024-03-23 20:38:17 浏览: 13
STMicroelectronics官方提供的STM32固件库中并没有DS18B20的驱动代码。但是,你可以参考以下示例代码,使用STM32的GPIO库和定时器库来实现DS18B20的驱动。
```c
#include "stm32f10x.h"
#define DS18B20_PIN GPIO_Pin_0
#define DS18B20_PORT GPIOA
uint8_t ds18b20_reset(void)
{
uint8_t presence;
GPIO_InitTypeDef GPIO_InitStructure;
// 配置GPIO为推挽输出模式
GPIO_InitStructure.GPIO_Pin = DS18B20_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
// 拉低总线至少480us
GPIO_ResetBits(DS18B20_PORT, DS18B20_PIN);
Delay_us(500);
// 释放总线, 读取DS18B20的存在脉冲
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
Delay_us(80);
presence = GPIO_ReadInputDataBit(DS18B20_PORT, DS18B20_PIN);
Delay_us(420);
return presence;
}
void ds18b20_write_bit(uint8_t bit)
{
GPIO_InitTypeDef GPIO_InitStructure;
// 配置GPIO为推挽输出模式
GPIO_InitStructure.GPIO_Pin = DS18B20_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
// 拉低总线至少1us
GPIO_ResetBits(DS18B20_PORT, DS18B20_PIN);
Delay_us(1);
// 发送bit, 持续至少60us
if (bit)
{
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
Delay_us(60);
}
else
{
Delay_us(60);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
}
}
uint8_t ds18b20_read_bit(void)
{
uint8_t bit;
GPIO_InitTypeDef GPIO_InitStructure;
// 配置GPIO为推挽输出模式
GPIO_InitStructure.GPIO_Pin = DS18B20_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
// 拉低总线至少1us
GPIO_ResetBits(DS18B20_PORT, DS18B20_PIN);
Delay_us(1);
// 释放总线, 读取bit, 持续至少14us
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(DS18B20_PORT, &GPIO_InitStructure);
Delay_us(14);
bit = GPIO_ReadInputDataBit(DS18B20_PORT, DS18B20_PIN);
Delay_us(46);
return bit;
}
void ds18b20_write_byte(uint8_t byte)
{
uint8_t i;
for (i = 0; i < 8; i++)
{
ds18b20_write_bit(byte & 0x01);
byte >>= 1;
}
}
uint8_t ds18b20_read_byte(void)
{
uint8_t i;
uint8_t byte = 0;
for (i = 0; i < 8; i++)
{
byte >>= 1;
if (ds18b20_read_bit())
byte |= 0x80;
}
return byte;
}
float ds18b20_read_temperature(void)
{
uint8_t presence;
uint8_t temp_l, temp_h;
float temp;
presence = ds18b20_reset();
if (presence)
{
ds18b20_write_byte(0xCC); // 跳过ROM操作
ds18b20_write_byte(0x44); // 启动温度转换
Delay_ms(750); // 等待转换完成
ds18b20_reset(); // 发送复位脉冲
ds18b20_write_byte(0xCC); // 跳过ROM操作
ds18b20_write_byte(0xBE); // 读取温度寄存器
temp_l = ds18b20_read_byte(); // 读取温度低字节
temp_h = ds18b20_read_byte(); // 读取温度高字节
// 计算温度值
temp = (float)(temp_h << 8 | temp_l) / 16.0f;
return temp;
}
else
{
return -273.15f; // DS18B20异常, 返回绝对零度
}
}
```
以上代码演示了如何使用STM32的GPIO库和定时器库来实现DS18B20的驱动。你需要调用ds18b20_read_temperature()函数来读取温度值。该函数会自动检测DS18B20的存在并进行温度转换。在调用该函数之前,你需要调用Delay_us()和Delay_ms()函数实现延时功能。
相关推荐
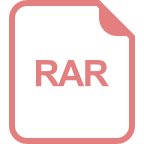
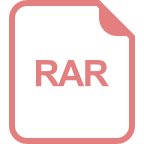
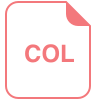
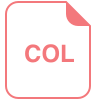
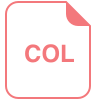
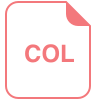
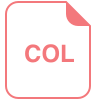









