b)挑战模式下,软件每次给出的成语,用户接龙由易到难(如,开始几轮可容易,到后来难度逐渐加大,但不能给无法接龙的成语。)这一板块具体的java代码
时间: 2024-03-14 21:46:13 浏览: 15
这里给出一个简单的实现示例,主要采用广度优先搜索算法。我们先定义一个成语类:
```java
public class Idiom {
private String word; // 成语
private int difficulty; // 难度得分
private List<Idiom> next; // 可接龙的下一个成语列表
public Idiom(String word, int difficulty) {
this.word = word;
this.difficulty = difficulty;
next = new ArrayList<>();
}
// 添加下一个可接龙的成语
public void addNext(Idiom idiom) {
next.add(idiom);
}
public String getWord() {
return word;
}
public int getDifficulty() {
return difficulty;
}
public List<Idiom> getNext() {
return next;
}
}
```
然后定义一个成语接龙类:
```java
public class IdiomGame {
private List<Idiom> allIdioms; // 全量成语列表
private List<Idiom> commonIdioms; // 常用成语列表
private List<Idiom> currentIdioms; // 当前可用成语列表
private List<Idiom> usedIdioms; // 已使用的成语列表
public IdiomGame() {
allIdioms = new ArrayList<>();
commonIdioms = new ArrayList<>();
currentIdioms = new ArrayList<>();
usedIdioms = new ArrayList<>();
}
// 从文件中读取成语列表并预处理
public void loadIdioms(String allPath, String commonPath) {
// 读取全量成语列表并预处理
// ...
// 读取常用成语列表并预处理
// ...
currentIdioms.addAll(commonIdioms);
}
// 根据当前成语生成下一个成语
public Idiom getNextIdiom(Idiom current, int round) {
List<Idiom> nextIdioms = current.getNext();
Collections.sort(nextIdioms, new Comparator<Idiom>() {
@Override
public int compare(Idiom o1, Idiom o2) {
return Integer.compare(o1.getDifficulty(), o2.getDifficulty());
}
});
int start = 0;
if (round > 1) {
start = (round - 2) * 5;
}
for (int i = start; i < nextIdioms.size(); i++) {
Idiom next = nextIdioms.get(i);
if (!usedIdioms.contains(next)) {
usedIdioms.add(next);
return next;
}
}
return null;
}
// 开始游戏
public void startGame(boolean isChallenge) {
int round = 1;
Idiom current = null;
while (true) {
if (current == null) {
System.out.println("请输入第" + round + "个成语:");
} else {
System.out.println("请回答第" + (round + 1) + "个成语:");
}
Scanner scanner = new Scanner(System.in);
String input = scanner.nextLine();
Idiom next = null;
if (current == null) {
for (Idiom idiom : currentIdioms) {
if (idiom.getWord().equals(input)) {
usedIdioms.add(idiom);
current = idiom;
next = getNextIdiom(current, round);
break;
}
}
} else {
for (Idiom idiom : current.getNext()) {
if (idiom.getWord().equals(input)) {
usedIdioms.add(idiom);
current = idiom;
next = getNextIdiom(current, round);
break;
}
}
}
if (next == null) {
System.out.println("答错了!");
break;
} else {
System.out.println("下一个成语:" + next.getWord());
if (isChallenge && round <= 10) {
System.out.println("提示:" + getNextIdiom(next, round + 1).getWord());
}
round++;
if (round > 10) {
System.out.println("您已完成挑战!");
break;
}
}
}
}
}
```
在主函数中,可以这样调用:
```java
public static void main(String[] args) {
IdiomGame game = new IdiomGame();
game.loadIdioms("all.txt", "common.txt");
game.startGame(true);
}
```
其中,`"all.txt"`和`"common.txt"`分别是全量成语和常用成语的词典文件路径,`true`表示选择挑战模式。在挑战模式下,每回合可以选择提示,提示的成语难度比下一个成语高一个等级。
相关推荐
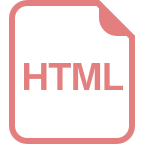
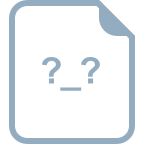
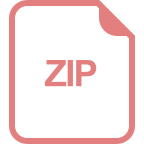














