JAVA用List实现高斯消元法
时间: 2024-04-29 18:21:19 浏览: 64
以下是使用Java的List实现高斯消元法的示例代码:
```java
import java.util.ArrayList;
import java.util.List;
public class GaussElimination {
public static void main(String[] args) {
List<List<Double>> matrix = new ArrayList<>();
matrix.add(new ArrayList<>(List.of(1.0, 2.0, -1.0, 2.0)));
matrix.add(new ArrayList<>(List.of(3.0, 6.0, -2.0, 9.0)));
matrix.add(new ArrayList<>(List.of(-1.0, -1.0, 2.0, 0.0)));
List<Double> result = new ArrayList<>(List.of(2.0, 4.0, 0.0));
List<Double> solution = solve(matrix, result);
System.out.println("Solution: " + solution);
}
public static List<Double> solve(List<List<Double>> matrix, List<Double> result) {
int n = matrix.size();
for (int i = 0; i < n; i++) {
// Find pivot row and swap
int maxRow = i;
for (int j = i + 1; j < n; j++) {
if (Math.abs(matrix.get(j).get(i)) > Math.abs(matrix.get(maxRow).get(i))) {
maxRow = j;
}
}
List<Double> temp = matrix.get(i);
matrix.set(i, matrix.get(maxRow));
matrix.set(maxRow, temp);
Double tempResult = result.get(i);
result.set(i, result.get(maxRow));
result.set(maxRow, tempResult);
// Reduce
for (int j = i + 1; j < n; j++) {
Double factor = matrix.get(j).get(i) / matrix.get(i).get(i);
matrix.get(j).set(i, 0.0);
for (int k = i + 1; k < n; k++) {
matrix.get(j).set(k, matrix.get(j).get(k) - factor * matrix.get(i).get(k));
}
result.set(j, result.get(j) - factor * result.get(i));
}
}
// Backward substitution
List<Double> solution = new ArrayList<>(n);
for (int i = n - 1; i >= 0; i--) {
Double sum = 0.0;
for (int j = i + 1; j < n; j++) {
sum += matrix.get(i).get(j) * solution.get(j);
}
solution.add(0, (result.get(i) - sum) / matrix.get(i).get(i));
}
return solution;
}
}
```
在这个示例中,我们首先定义了一个4x4的矩阵和一个长度为3的结果向量,以进行高斯消元法的演示。我们使用了Java的List来表示矩阵和向量,并使用嵌套的List来表示行和列。
在`solve()`方法中,我们使用了高斯消元法的标准算法。我们首先找到一个主元素并将其所在的行与第i行进行交换,然后将主元素下方的所有元素消为0。然后,我们使用反向代换来计算方程组的解。
最后,我们输出结果向量作为解。请注意,这个示例仅适用于方程组有唯一解的情况。如果方程组具有无穷多解或无解,则需要使用其他算法。
阅读全文
相关推荐

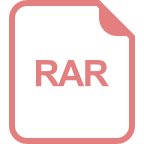










