根据以下要求使用asp.net代码实现并给出包含页面设计的全部代码:在首次登录后,能够将登录信息写入,并可以选择保存登录信息至用户计算机的cookie中,当再次登录时,将用户计算机中的Cookie信息读出并显示,以备用户选择使用,能够利用读出Cookie中的信息来直接登录站点。
时间: 2023-09-13 16:08:23 浏览: 80
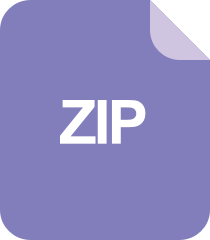
asp.net登录页面设计

以下是ASP.NET页面代码及其设计:
```html
<%@ Page Language="C#" %>
<!DOCTYPE html>
<html>
<head>
<title>Cookie 示例</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h1>登录</h1>
<asp:Label ID="lblMessage" runat="server"></asp:Label><br />
用户名:<asp:TextBox ID="txtUsername" runat="server"></asp:TextBox><br />
密码:<asp:TextBox ID="txtPassword" runat="server" TextMode="Password"></asp:TextBox><br />
<asp:CheckBox ID="chkRememberMe" runat="server" Text="记住我" /><br />
<asp:Button ID="btnLogin" runat="server" Text="登录" OnClick="btnLogin_Click" />
</div>
</form>
</body>
</html>
```
在这个页面中,我们有一个表单 `form1`,其中包含了一个用户名输入框 `txtUsername`、一个密码输入框 `txtPassword`、一个记住我复选框 `chkRememberMe` 和一个登录按钮 `btnLogin`。在用户输入用户名和密码后点击登录按钮,将触发 `btnLogin_Click` 事件。
接下来是ASP.NET后端代码:
```csharp
using System;
using System.Web;
public partial class Login : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
// 检查是否有保存的Cookie
HttpCookie cookie = Request.Cookies["LoginInfo"];
if (cookie != null)
{
txtUsername.Text = cookie.Values["Username"];
txtPassword.Text = cookie.Values["Password"];
chkRememberMe.Checked = true;
}
}
}
protected void btnLogin_Click(object sender, EventArgs e)
{
string username = txtUsername.Text;
string password = txtPassword.Text;
// 在这里验证用户名和密码是否正确
if (chkRememberMe.Checked)
{
// 保存Cookie
HttpCookie cookie = new HttpCookie("LoginInfo");
cookie.Values.Add("Username", username);
cookie.Values.Add("Password", password);
cookie.Expires = DateTime.Now.AddDays(7); // 有效期为7天
Response.Cookies.Add(cookie);
}
else
{
// 删除Cookie
Response.Cookies["LoginInfo"].Expires = DateTime.Now.AddDays(-1);
}
// 在这里进行登录操作
// ...
}
}
```
在这段代码中,我们首先在 `Page_Load` 事件中检查是否有保存的Cookie,若有则将用户名和密码分别填充到相应的输入框中,并将记住我复选框设为选中状态。
在 `btnLogin_Click` 事件中,我们首先获取用户输入的用户名和密码,并进行验证。如果用户勾选了记住我复选框,则创建一个新的Cookie,将用户名和密码分别添加到该Cookie的 `Values` 集合中,并将其过期时间设置为7天后;否则,直接删除名为 “LoginInfo” 的Cookie。最后,我们可以在这里进行登录操作,比如跳转到另一个页面。
需要注意的是,从安全性角度考虑,我们不应该直接将用户的密码存储在Cookie中,而应该使用加密算法对其进行加密。此外,用户的Cookie信息可能会被修改或伪造,因此我们在使用Cookie时也需要进行一些安全性验证。
阅读全文
相关推荐
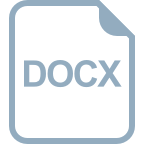

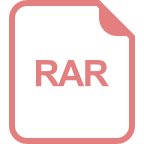
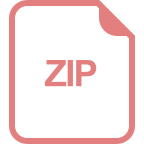
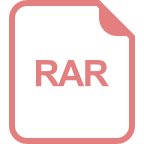
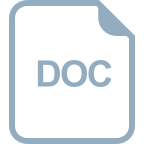
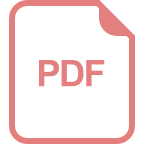
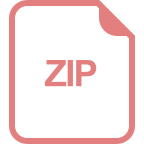
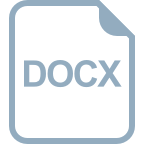
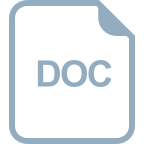
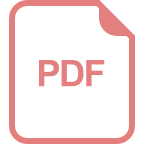
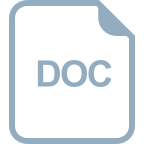
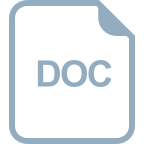
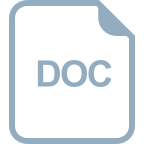
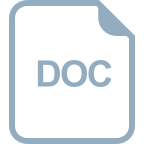
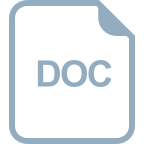
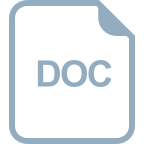