-输入 10个学生 5门课的成绩,分别用函数实现下列功能: (1)计算每个学生的平均分:+ (2)计算每门课的平均分:4
时间: 2023-06-06 22:04:25 浏览: 86
好的,我可以回答这个问题。
对于第一个功能,可以使用以下代码实现:
```
#include <stdio.h>
void calcAvgPerStu(int scores[][5], int stuNum) {
for (int i = 0; i < stuNum; i++) {
int sum = 0;
for (int j = 0; j < 5; j++) {
sum += scores[i][j];
}
printf("Student %d's average score is: %.2f\n", i+1, sum / 5.0);
}
}
int main() {
int scores[10][5]; // 存储学生的成绩
for (int i = 0; i < 10; i++) {
printf("Please enter the scores of Student %d (separated by space): ", i+1);
for (int j = 0; j < 5; j++) {
scanf("%d", &scores[i][j]);
}
}
calcAvgPerStu(scores, 10); // 计算每个学生的平均分
return 0;
}
```
对于第二个功能,可以使用以下代码实现:
```
#include <stdio.h>
void calcAvgPerSub(int scores[][5]) {
for (int i = 0; i < 5; i++) {
int sum = 0;
for (int j = 0; j < 10; j++) {
sum += scores[j][i];
}
printf("Subject %d's average score is: %.2f\n", i+1, sum / 10.0);
}
}
int main() {
int scores[10][5]; // 存储学生的成绩
for (int i = 0; i < 10; i++) {
printf("Please enter the scores of Student %d (separated by space): ", i+1);
for (int j = 0; j < 5; j++) {
scanf("%d", &scores[i][j]);
}
}
calcAvgPerSub(scores); // 计算每门课的平均分
return 0;
}
```
以上是使用 C 语言实现的代码,可以通过输入 10 个学生 5 门课的成绩,计算每个学生的平均分和每门课的平均分。希望能对您有所帮助。
相关推荐
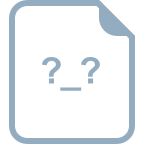
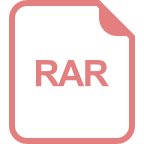
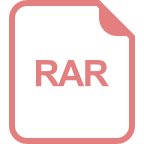














