Python 面向对象推箱子小游戏
时间: 2023-07-02 14:20:14 浏览: 214
好的,我可以为你提供一个 Python 面向对象推箱子小游戏的例子,帮助你更好地理解面向对象编程的应用。在这个小游戏中,玩家需要将箱子推到指定位置,才能过关。
```python
class Box:
def __init__(self, x, y):
self.x = x
self.y = y
def move(self, dx, dy):
self.x += dx
self.y += dy
def is_at(self, x, y):
return self.x == x and self.y == y
class Map:
def __init__(self, width, height):
self.width = width
self.height = height
self.player = Box(0, 0)
self.boxes = [Box(3, 2), Box(4, 3)]
self.destinations = [(2, 2), (3, 3)]
def draw(self):
for y in range(self.height):
for x in range(self.width):
if self.player.is_at(x, y):
print("P", end="")
elif (x, y) in self.destinations:
if any(box.is_at(x, y) for box in self.boxes):
print("B", end="")
else:
print("D", end="")
elif any(box.is_at(x, y) for box in self.boxes):
print("B", end="")
else:
print(".", end="")
print()
def move_player(self, dx, dy):
new_x = self.player.x + dx
new_y = self.player.y + dy
if (new_x, new_y) in self.destinations:
self.player.move(dx, dy)
self.draw()
print("恭喜你,过关了!")
elif any(box.is_at(new_x, new_y) for box in self.boxes):
for box in self.boxes:
if box.is_at(new_x, new_y):
new_box_x = box.x + dx
new_box_y = box.y + dy
if (new_box_x, new_box_y) in self.destinations:
box.move(dx, dy)
self.player.move(dx, dy)
self.draw()
print("恭喜你,过关了!")
break
elif not any(b.is_at(new_box_x, new_box_y) for b in self.boxes):
box.move(dx, dy)
self.player.move(dx, dy)
self.draw()
break
else:
print("箱子被挡住了!")
break
elif 0 <= new_x < self.width and 0 <= new_y < self.height:
self.player.move(dx, dy)
self.draw()
else:
print("不能走出边界!")
game = Map(6, 6)
game.draw()
while True:
direction = input("请输入方向(上下左右):")
if direction == "上":
game.move_player(0, -1)
elif direction == "下":
game.move_player(0, 1)
elif direction == "左":
game.move_player(-1, 0)
elif direction == "右":
game.move_player(1, 0)
else:
print("输入有误,请重新输入!")
```
在这个例子中,我们定义了两个类 `Box` 和 `Map`。`Box` 类表示一个箱子,包括其所在位置和移动方法;`Map` 类表示整个游戏地图,包括地图大小、玩家位置、箱子位置、目的地位置和移动方法。在 `Map` 类中,我们使用了列表来存储多个箱子和多个目的地位置,并使用 `draw` 方法来绘制地图。在移动方法中,我们处理了玩家移动、箱子移动和过关等情况,使用了循环、条件语句和列表推导式等技巧。
在主程序中,我们创建了一个 `Map` 对象,并通过 `while` 循环来不断获取玩家的输入,并调用 `move_player` 方法进行移动。当玩家将所有箱子推到目的地时,循环退出。
这个小游戏虽然稍微复杂一些,但是也非常有趣,可以帮助你更好地理解面向对象编程的应用。如果你想进一步提高自己的编程水平,可以尝试实现更加复杂的小游戏,例如扫雷、俄罗斯方块等。
阅读全文
相关推荐
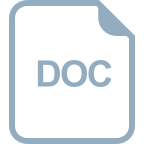


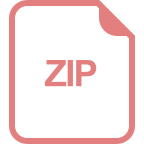
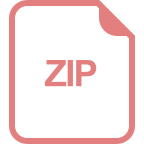
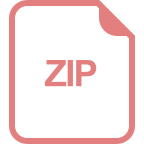
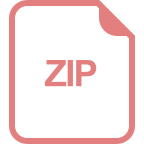
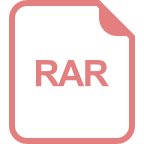
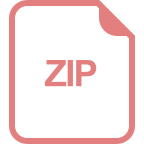
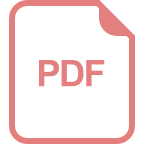
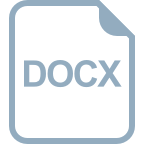
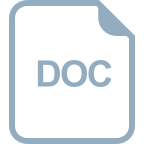
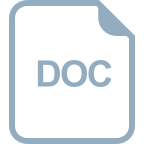
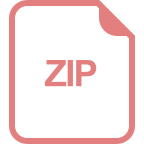