编写一个ATM取款机小程序
时间: 2023-05-26 19:01:18 浏览: 251
A:
首先,在python中创建一个ATM类,该类将包含以下方法:
1. __init__: 用于初始化ATM类对象的构造函数,接受账户余额作为参数。
2. check_balance: 检查账户余额的方法。
3. withdraw: 用于从账户中提取指定数量的现金的方法。
4. deposit: 用于向账户中存入现金的方法。
下面是一个简单的ATM类实现:
```python
class ATM:
def __init__(self, balance):
self.balance = balance
def check_balance(self):
print("Your account balance is: $", self.balance)
def withdraw(self, amount):
if amount > self.balance:
print("Insufficient funds in your account!")
else:
self.balance -= amount
print("$", amount, "withdrawn. Remaining balance is: $", self.balance)
def deposit(self, amount):
self.balance += amount
print("$", amount, "deposited. Updated balance is: $", self.balance)
```
现在,我们可以在主函数中使用该类来编写ATM小程序:
```python
balance = 1000
myATM = ATM(balance)
print("Welcome to the ATM!")
while True:
print("\nSelect an option:")
print("1. Check balance")
print("2. Withdraw")
print("3. Deposit")
print("4. Exit")
choice = int(input("Enter your choice: "))
if choice == 1:
myATM.check_balance()
elif choice == 2:
amount = int(input("Enter the amount to be withdrawn: $"))
myATM.withdraw(amount)
elif choice == 3:
amount = int(input("Enter the amount to be deposited: $"))
myATM.deposit(amount)
elif choice == 4:
print("Thank you for using the ATM!")
break
else:
print("Invalid choice! Please select a valid option.")
```
该程序将显示一个欢迎消息,接着会一直询问你想做什么,直到你选择退出为止。根据你选择的选项,它会调用相应的ATM类方法来执行特定的操作。
阅读全文
相关推荐


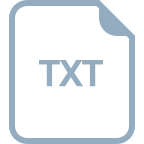
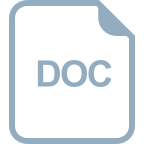
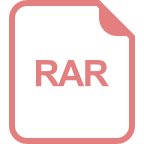
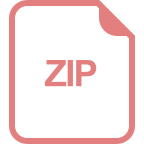
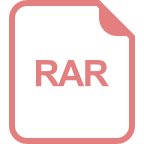
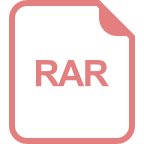
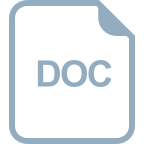
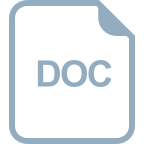
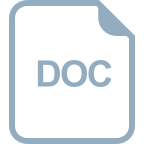
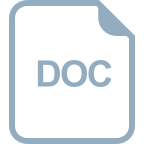