C语言计算线程负责计算(1到100的累加,每加一个数后打印一次),结果为偶数由打印线程1打印,奇数由打印线程2打印; 主进程等待子线程退出
时间: 2024-05-10 11:15:58 浏览: 14
以下是一个实现这个功能的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define MAX_NUM 100
int sum = 0;
pthread_mutex_t mutex;
pthread_cond_t even_cond, odd_cond;
void *calc_thread(void *arg) {
for (int i = 1; i <= MAX_NUM; i++) {
pthread_mutex_lock(&mutex);
sum += i;
printf("sum: %d\n", sum);
if (sum % 2 == 0) {
pthread_cond_signal(&even_cond);
} else {
pthread_cond_signal(&odd_cond);
}
pthread_mutex_unlock(&mutex);
}
pthread_exit(NULL);
}
void *print_even_thread(void *arg) {
while (1) {
pthread_mutex_lock(&mutex);
pthread_cond_wait(&even_cond, &mutex);
printf("Even: %d\n", sum);
pthread_mutex_unlock(&mutex);
if (sum == MAX_NUM * (MAX_NUM + 1) / 2) {
break;
}
}
pthread_exit(NULL);
}
void *print_odd_thread(void *arg) {
while (1) {
pthread_mutex_lock(&mutex);
pthread_cond_wait(&odd_cond, &mutex);
printf("Odd: %d\n", sum);
pthread_mutex_unlock(&mutex);
if (sum == MAX_NUM * (MAX_NUM + 1) / 2) {
break;
}
}
pthread_exit(NULL);
}
int main() {
pthread_t calc_tid, print_even_tid, print_odd_tid;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&even_cond, NULL);
pthread_cond_init(&odd_cond, NULL);
pthread_create(&calc_tid, NULL, calc_thread, NULL);
pthread_create(&print_even_tid, NULL, print_even_thread, NULL);
pthread_create(&print_odd_tid, NULL, print_odd_thread, NULL);
pthread_join(calc_tid, NULL);
pthread_join(print_even_tid, NULL);
pthread_join(print_odd_tid, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&even_cond);
pthread_cond_destroy(&odd_cond);
return 0;
}
```
这个程序使用了三个线程:计算线程、偶数打印线程和奇数打印线程。计算线程负责计算1到100的累加,并且每加一个数后打印一次,然后根据计算结果是偶数还是奇数发出条件变量信号。偶数打印线程和奇数打印线程分别等待偶数条件变量和奇数条件变量的信号,并且打印计算结果。当计算线程计算完毕后,所有线程都退出。
相关推荐
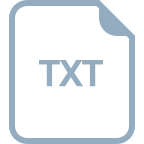














