以下是哈夫曼树的创建,怎么把节点值和权值由键盘输入改成从文件中读取信息://创造哈夫曼树 void CreateHuffmanTree(HuffmanTree &HT, int num) { int m,f; char c; m = 2 * num - 1; HT = new HTNode[m + 1]; //分配空间 for (int i = 1; i <= m; i++) //初始化 { HT[i].parent = 0; HT[i].lchild = 0; HT[i].rchild = 0; } cout << "请输入每个数据及其权值:" << endl; for (int i = 1; i <= num; i++) { printf("输入第%d个元素的=>\n",i); printf("\t结点值:"); scanf("%c",&c); HT[i].data=getchar(); printf("\t权值:"); scanf("%d",&f); HT[i].weight=f; //scanf("%d",HT[i].weight) ; //HT[i].data=n; //scanf("%[^\n]",HT[i].data); // cin >> HT[i].weight; // cin>>HT[i].data; } for (int i = num + 1; i <= m; i++) //构建哈夫曼树 { MIN min; min=Select(HT,i-1); //选择二叉树 HT[min.s1].parent = i; HT[min.s2].parent = i; HT[i].lchild = min.s1; HT[min.s1].num = "0"; HT[i].rchild = min.s2; HT[min.s2].num = "1"; HT[i].weight = HT[min.s1].weight + HT[min.s2].weight; HT[i].data = -1; } putlorinnum(HT, m); for (int i = 1; i <= m; i++) //进行每个字符哈夫曼码的输出 { if(HT[i].data != -1) { cout<<HT[i].data<<" 权重为"<<HT[i].weight<<" ,哈夫曼码为:"<<HT[i].num<<endl; cout<<endl; } } }
时间: 2024-01-07 13:05:17 浏览: 26
可以使用文件输入流来读取信息,具体操作如下:
1. 在头文件中添加 `#include<fstream>`,使用文件输入输出流需要包含这个头文件。
2. 打开文件,将文件中的信息读入到程序中。
```c++
ifstream infile("filename.txt"); //打开文件
if (!infile) //文件打开失败
{
cout << "文件打开失败!" << endl;
exit(1);
}
for (int i = 1; i <= num; i++)
{
printf("输入第%d个元素的=>\n",i);
printf("\t结点值:");
infile>>c;
HT[i].data = c;
printf("\t权值:");
infile>>f;
HT[i].weight = f;
}
infile.close(); //关闭文件
```
3. 将输入流对象作为参数传递给函数,即修改函数声明为:
```c++
void CreateHuffmanTree(HuffmanTree &HT, int num, ifstream &infile)
```
4. 在主函数中调用时,传递文件输入流对象作为参数:
```c++
ifstream infile("filename.txt"); // 打开文件
CreateHuffmanTree(HT, num, infile); // 调用函数
infile.close(); // 关闭文件
```
相关问题
对以下代码进行细致注释#pragma once #include <map> #include <iomanip> //哈夫曼树存储结构 struct HuffmanNode { int weight; //结点字符和权值 int parent, lchild, rchild; }; class Huffman { private: map<char, int> code; map<string, char> sc_code; map<char, string> cs_code; const int MAXWEIGHT = 2147483647; HuffmanNode* hf = NULL; int N = 0; public: //无参构造 Huffman(); //初始化 void Initialization(); //选择 void select(HuffmanNode*& hf, int n, int& s1, int& s2); //创建Huffman树 void createHuffmanTree(HuffmanNode*& hf, int n); //Huffman编码实现 char** createHuffmanCode(HuffmanNode*& hf, int n); //编码 void Encoding(); //译码 void Decoding(); //印代码文件 void Print(); //印哈夫曼树 void TreePrinting(); };
// 防止头文件重复引用
#pragma once
// 使用map需要引入的头文件
#include <map>
// 用于输出格式化的头文件
#include <iomanip>
// 定义哈夫曼树的结构体
struct HuffmanNode {
int weight; // 结点字符和权值
int parent, lchild, rchild; // 结点父节点、左孩子节点和右孩子节点
};
// 定义哈夫曼编码的类
class Huffman {
private:
// 原始字符 -> 权值的映射
map<char, int> code;
// 压缩后的二进制位 -> 原始字符的映射
map<string, char> sc_code;
// 原始字符 -> 压缩后的二进制位的映射
map<char, string> cs_code;
// 定义最大权值
const int MAXWEIGHT = 2147483647;
// 定义哈夫曼树的指针
HuffmanNode* hf = NULL;
// 定义哈夫曼树的结点数目
int N = 0;
public:
// 无参构造函数
Huffman();
// 初始化哈夫曼编码
void Initialization();
// 选择权值最小的两个结点
void select(HuffmanNode*& hf, int n, int& s1, int& s2);
// 创建哈夫曼树
void createHuffmanTree(HuffmanNode*& hf, int n);
// 实现哈夫曼编码
char** createHuffmanCode(HuffmanNode*& hf, int n);
// 编码
void Encoding();
// 译码
void Decoding();
// 输出压缩后的代码文件
void Print();
// 输出哈夫曼树的结构
void TreePrinting();
};
用c语言数据结构编写程序,输入结点个数及各结点对应的权值,创建哈夫曼树,生成哈夫曼编码并输出。
以下是用C语言数据结构编写的哈夫曼树程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_NODE 100 // 最大结点数
// 哈夫曼树结点结构体
typedef struct {
int weight; // 权值
int parent, lchild, rchild; // 父结点、左子结点、右子结点的下标
} HuffmanNode;
// 哈夫曼编码结构体
typedef struct {
char ch; // 字符
char code[MAX_NODE]; // 编码
} HuffmanCode;
// 选择权值最小的两个结点
void selectMinNodes(HuffmanNode *nodes, int n, int *min1, int *min2) {
int i, j;
*min1 = *min2 = -1;
for (i = 0; i < n; i++) {
if (nodes[i].parent == -1) { // 未被选择
if (*min1 == -1 || nodes[i].weight < nodes[*min1].weight) {
*min2 = *min1;
*min1 = i;
} else if (*min2 == -1 || nodes[i].weight < nodes[*min2].weight) {
*min2 = i;
}
}
}
}
// 创建哈夫曼树
void createHuffmanTree(HuffmanNode *nodes, int n) {
int i, min1, min2;
for (i = 0; i < n - 1; i++) {
selectMinNodes(nodes, n + i, &min1, &min2);
nodes[min1].parent = n + i;
nodes[min2].parent = n + i;
nodes[n + i].weight = nodes[min1].weight + nodes[min2].weight;
nodes[n + i].lchild = min1;
nodes[n + i].rchild = min2;
}
}
// 生成哈夫曼编码
void generateHuffmanCode(HuffmanNode *nodes, int n, HuffmanCode *codes) {
int i, j, parent, current;
char code[MAX_NODE + 1];
code[n] = '\0';
for (i = 0; i < n; i++) {
codes[i].ch = i; // 字符
current = i;
parent = nodes[current].parent;
while (parent != -1) { // 从叶子结点向上走到根结点
if (nodes[parent].lchild == current) {
code[--n] = '0';
} else {
code[--n] = '1';
}
current = parent;
parent = nodes[current].parent;
}
strcpy(codes[i].code, &code[n]); // 复制编码
}
}
int main() {
int n, i;
HuffmanNode nodes[MAX_NODE];
HuffmanCode codes[MAX_NODE];
printf("请输入结点个数:");
scanf("%d", &n);
printf("请输入各结点的权值:");
for (i = 0; i < n; i++) {
scanf("%d", &nodes[i].weight);
nodes[i].parent = -1;
nodes[i].lchild = -1;
nodes[i].rchild = -1;
}
createHuffmanTree(nodes, n);
generateHuffmanCode(nodes, n, codes);
printf("哈夫曼编码表:\n");
for (i = 0; i < n; i++) {
printf("%c: %s\n", codes[i].ch, codes[i].code);
}
return 0;
}
```
程序的思路如下:
1. 定义哈夫曼树结点结构体和哈夫曼编码结构体;
2. 输入结点个数和各结点的权值;
3. 创建哈夫曼树,即重复选择权值最小的两个结点,合并为一个新的结点,直到只剩下一个根结点;
4. 生成哈夫曼编码,即从每个叶子结点向上走到根结点,记录路径上的 '0' 和 '1',得到对应的编码;
5. 输出哈夫曼编码表。
注意:程序中有一个假设,即每个结点对应的字符就是该字符在 ASCII 码表中的编码。如果需要处理其他字符集,需要修改程序。
相关推荐
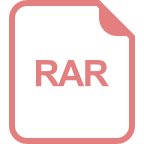
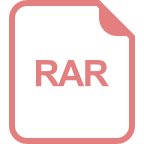
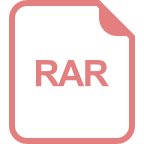












