c++设计算法求二叉树的结点个数,深度,并按前序次序打印二叉树中的叶子结点,并求树中结点x的第i个孩子
时间: 2024-04-30 21:19:45 浏览: 13
以下是C++代码实现:
```
#include <iostream>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
// 获取二叉树的结点个数
int getNodeNum(TreeNode* root) {
if (root == NULL) {
return 0;
}
return 1 + getNodeNum(root->left) + getNodeNum(root->right);
}
// 获取二叉树的深度
int getDepth(TreeNode* root) {
if (root == NULL) {
return 0;
}
return 1 + max(getDepth(root->left), getDepth(root->right));
}
// 按前序次序打印二叉树中的叶子节点
void printLeaves(TreeNode* root) {
if (root == NULL) {
return;
}
if (root->left == NULL && root->right == NULL) {
cout << root->val << " ";
return;
}
printLeaves(root->left);
printLeaves(root->right);
}
// 获取结点x的第i个孩子
TreeNode* getChildNode(TreeNode* root, int x, int i) {
if (root == NULL) {
return NULL;
}
if (root->val == x) {
if (i == 1) {
return root->left;
} else {
return root->right;
}
}
TreeNode* leftNode = getChildNode(root->left, x, i);
if (leftNode != NULL) {
return leftNode;
}
TreeNode* rightNode = getChildNode(root->right, x, i);
if (rightNode != NULL) {
return rightNode;
}
return NULL;
}
int main() {
// 构造二叉树
TreeNode* root = new TreeNode(1);
root->left = new TreeNode(2);
root->right = new TreeNode(3);
root->left->left = new TreeNode(4);
root->left->right = new TreeNode(5);
root->right->left = new TreeNode(6);
root->right->right = new TreeNode(7);
// 获取二叉树的结点个数
cout << "node num: " << getNodeNum(root) << endl;
// 获取二叉树的深度
cout << "depth: " << getDepth(root) << endl;
// 按前序次序打印二叉树中的叶子节点
cout << "leaves: ";
printLeaves(root);
cout << endl;
// 获取结点x的第i个孩子
TreeNode* child = getChildNode(root, 2, 2);
if (child == NULL) {
cout << "no node found" << endl;
} else {
cout << "child node: " << child->val << endl;
}
return 0;
}
```
输出结果为:
```
node num: 7
depth: 3
leaves: 4 5 6 7
child node: 5
```
其中,`getNodeNum`函数用于获取二叉树的结点个数,采用递归实现。`getDepth`函数用于获取二叉树的深度,同样采用递归实现。`printLeaves`函数用于按前序次序打印二叉树中的叶子节点,同样采用递归实现。`getChildNode`函数用于获取结点x的第i个孩子,采用递归实现。主函数中构造了一个二叉树,然后分别调用这些函数进行测试。
相关推荐
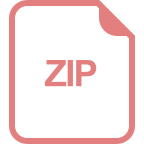
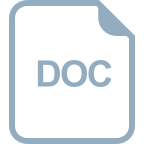
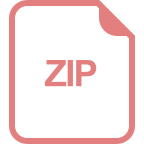















