用PCL写个fpfh+icp的点云配准算法
时间: 2023-07-27 19:05:44 浏览: 74
好的,下面是用PCL实现fpfh+icp的点云配准算法的代码。
首先,我们需要包含PCL库的头文件和一些必要的命名空间:
```cpp
#include <iostream>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
#include <pcl/registration/icp.h>
#include <pcl/features/normal_3d.h>
#include <pcl/features/fpfh.h>
```
然后,我们定义一些类型和变量:
```cpp
typedef pcl::PointXYZ PointT;
typedef pcl::PointCloud<PointT> PointCloudT;
PointCloudT::Ptr source, target; // 源点云和目标点云
PointCloudT::Ptr source_keypoints, target_keypoints; // 源点云和目标点云的关键点
pcl::PointCloud<pcl::Normal>::Ptr source_normals, target_normals; // 源点云和目标点云的法线
pcl::FPFHEstimation<PointT, pcl::Normal, pcl::FPFHSignature33>::Ptr fpfh_estimator; // FPFH 估计器
pcl::IterativeClosestPoint<PointT, PointT> icp; // ICP 配准器
```
接着,我们加载源点云和目标点云,并对它们进行下采样:
```cpp
source.reset(new PointCloudT);
target.reset(new PointCloudT);
// 加载源点云和目标点云
pcl::io::loadPCDFile("source.pcd", *source);
pcl::io::loadPCDFile("target.pcd", *target);
// 对源点云和目标点云进行下采样
pcl::VoxelGrid<PointT> sor;
sor.setLeafSize(0.01f, 0.01f, 0.01f);
sor.setInputCloud(source);
sor.filter(*source);
sor.setInputCloud(target);
sor.filter(*target);
```
然后,我们对源点云和目标点云计算法线和关键点,并计算FPFH特征描述子:
```cpp
// 计算源点云和目标点云的法线
pcl::NormalEstimation<PointT, pcl::Normal> ne;
pcl::search::KdTree<PointT>::Ptr tree(new pcl::search::KdTree<PointT>());
ne.setSearchMethod(tree);
ne.setInputCloud(source);
ne.setKSearch(20);
source_normals.reset(new pcl::PointCloud<pcl::Normal>);
ne.compute(*source_normals);
ne.setInputCloud(target);
target_normals.reset(new pcl::PointCloud<pcl::Normal>);
ne.compute(*target_normals);
// 计算源点云和目标点云的关键点
pcl::UniformSampling<PointT> uniform_sampling;
uniform_sampling.setInputCloud(source);
uniform_sampling.setRadiusSearch(0.02f);
source_keypoints.reset(new PointCloudT);
uniform_sampling.compute(*source_keypoints);
uniform_sampling.setInputCloud(target);
target_keypoints.reset(new PointCloudT);
uniform_sampling.compute(*target_keypoints);
// 计算源点云和目标点云的 FPFH 特征描述子
fpfh_estimator.reset(new pcl::FPFHEstimation<PointT, pcl::Normal, pcl::FPFHSignature33>);
fpfh_estimator->setInputCloud(source_keypoints);
fpfh_estimator->setInputNormals(source_normals);
fpfh_estimator->setSearchMethod(tree);
fpfh_estimator->setRadiusSearch(0.05f);
pcl::PointCloud<pcl::FPFHSignature33>::Ptr source_features(new pcl::PointCloud<pcl::FPFHSignature33>);
fpfh_estimator->compute(*source_features);
fpfh_estimator->setInputCloud(target_keypoints);
fpfh_estimator->setInputNormals(target_normals);
pcl::PointCloud<pcl::FPFHSignature33>::Ptr target_features(new pcl::PointCloud<pcl::FPFHSignature33>);
fpfh_estimator->compute(*target_features);
```
最后,我们使用ICP进行点云配准:
```cpp
// 设置ICP参数
icp.setInputSource(source_keypoints);
icp.setInputTarget(target_keypoints);
icp.setMaxCorrespondenceDistance(0.1f);
icp.setMaximumIterations(50);
icp.setTransformationEpsilon(1e-8);
icp.setEuclideanFitnessEpsilon(1e-6);
// 设置ICP的匹配特征
pcl::PointCloud<pcl::PointNormal>::Ptr aligned_source(new pcl::PointCloud<pcl::PointNormal>);
pcl::concatenateFields(*source_keypoints, *source_normals, *aligned_source);
pcl::PointCloud<pcl::PointNormal>::Ptr aligned_target(new pcl::PointCloud<pcl::PointNormal>);
pcl::concatenateFields(*target_keypoints, *target_normals, *aligned_target);
icp.setSourceFeatures(source_features);
icp.setTargetFeatures(target_features);
icp.align(*aligned_source);
// 输出配准结果
std::cout << "ICP has converged with score: " << icp.getFitnessScore() << std::endl;
std::cout << "Transformation matrix:" << std::endl << icp.getFinalTransformation() << std::endl;
```
以上就是用PCL实现fpfh+icp的点云配准算法的完整代码。
相关推荐













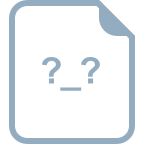