java集合实现扣费后查看账户余额
时间: 2023-11-11 17:10:03 浏览: 104
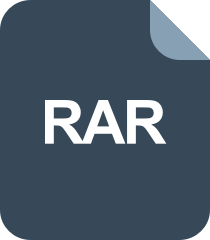
java web银行余额查询系统

假设你有一个账户类 Account,其中包含了账户余额 balance 属性和扣费方法 deductFee(),那么你可以利用 Java 集合中的 List 来存储多个账户对象。具体实现步骤如下:
1. 定义 Account 类
```java
public class Account {
private double balance;
public Account(double balance) {
this.balance = balance;
}
public double getBalance() {
return balance;
}
public void deductFee(double fee) {
balance -= fee;
}
}
```
2. 创建多个账户对象并添加到 List 中
```java
List<Account> accounts = new ArrayList<>();
accounts.add(new Account(1000));
accounts.add(new Account(2000));
accounts.add(new Account(3000));
```
3. 执行扣费操作并查看账户余额
```java
// 扣除第一个账户的 100 元费用
accounts.get(0).deductFee(100);
// 查看第一个账户的余额
System.out.println("Account 1 balance: " + accounts.get(0).getBalance());
// 扣除所有账户的 200 元费用
for (Account account : accounts) {
account.deductFee(200);
}
// 遍历所有账户并打印余额
for (int i = 0; i < accounts.size(); i++) {
System.out.println("Account " + (i+1) + " balance: " + accounts.get(i).getBalance());
}
```
输出结果为:
```
Account 1 balance: 900.0
Account 1 balance: 700.0
Account 2 balance: 1800.0
Account 3 balance: 2800.0
```
阅读全文
相关推荐
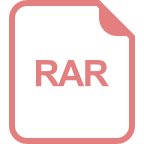
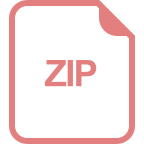
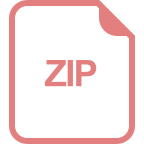
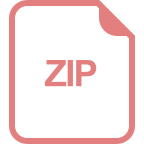
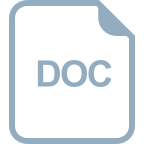
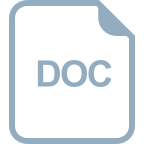
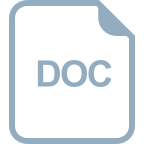
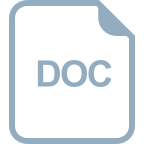
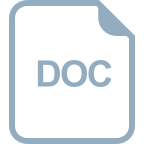
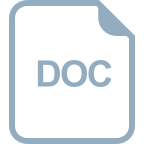
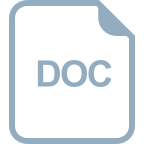
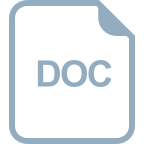
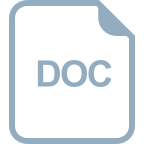
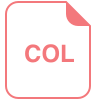
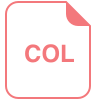
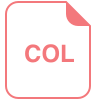
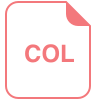
