用QT写一个G代码解释器
时间: 2024-01-28 13:05:38 浏览: 137
好的,下面是一个简单的G代码解释器的QT实现:
1. 创建一个新的QT项目,选择“应用程序”模板。
2. 在项目中添加一个新的类,命名为“GCodeInterpreter”。
3. 在GCodeInterpreter类中添加以下变量和函数:
```c++
private:
QString m_gCode; // 存储G代码
int m_lineNumber; // 当前执行的行号
QMap<QString, double> m_variables; // 存储变量及其值的映射表
double getVariableValue(const QString& variableName); // 获取变量的值
void setVariableValue(const QString& variableName, double value); // 设置变量的值
void executeCurrentLine(); // 执行当前行
```
4. 实现getVariableValue和setVariableValue函数,用于获取和设置变量的值:
```c++
double GCodeInterpreter::getVariableValue(const QString& variableName)
{
if (m_variables.contains(variableName)) {
return m_variables[variableName];
} else {
return 0.0;
}
}
void GCodeInterpreter::setVariableValue(const QString& variableName, double value)
{
m_variables[variableName] = value;
}
```
5. 实现executeCurrentLine函数,用于执行当前行的G代码:
```c++
void GCodeInterpreter::executeCurrentLine()
{
QString line = m_gCode.split('\n')[m_lineNumber - 1].trimmed(); // 获取当前行的G代码
// 解析G代码
QStringList parts = line.split(' ');
QString command = parts[0].toUpper();
QStringList arguments = parts.mid(1);
// 根据G代码命令执行相应的操作
if (command == "G00" || command == "G01") {
// 移动
double x = getVariableValue("X");
double y = getVariableValue("Y");
double z = getVariableValue("Z");
for (const QString& argument : arguments) {
QStringList argParts = argument.split('=');
QString argName = argParts[0].toUpper();
double argValue = argParts[1].toDouble();
if (argName == "X") {
x = argValue;
} else if (argName == "Y") {
y = argValue;
} else if (argName == "Z") {
z = argValue;
}
}
// 执行移动操作
qDebug() << "Moving to (" << x << ", " << y << ", " << z << ")";
} else if (command == "G02" || command == "G03") {
// 圆弧
double x = getVariableValue("X");
double y = getVariableValue("Y");
double z = getVariableValue("Z");
double i = getVariableValue("I");
double j = getVariableValue("J");
double k = getVariableValue("K");
double radius = qSqrt(qPow(i, 2) + qPow(j, 2) + qPow(k, 2));
for (const QString& argument : arguments) {
QStringList argParts = argument.split('=');
QString argName = argParts[0].toUpper();
double argValue = argParts[1].toDouble();
if (argName == "X") {
x = argValue;
} else if (argName == "Y") {
y = argValue;
} else if (argName == "Z") {
z = argValue;
} else if (argName == "I") {
i = argValue;
} else if (argName == "J") {
j = argValue;
} else if (argName == "K") {
k = argValue;
radius = qSqrt(qPow(i, 2) + qPow(j, 2) + qPow(k, 2));
}
}
// 执行圆弧操作
qDebug() << "Drawing arc with radius" << radius << "from (" << x << ", " << y << ", " << z << ")";
} else if (command == "M02" || command == "M30") {
// 停止
qDebug() << "Stopping execution";
} else if (command == "M03" || command == "M04") {
// 开启刀具
qDebug() << "Turning on tool";
} else if (command == "M05") {
// 关闭刀具
qDebug() << "Turning off tool";
} else if (command == "M06") {
// 更换刀具
qDebug() << "Changing tool";
} else if (command == "M08") {
// 开启冷却液
qDebug() << "Turning on coolant";
} else if (command == "M09") {
// 关闭冷却液
qDebug() << "Turning off coolant";
}
m_lineNumber++; // 前往下一行
}
```
6. 在GCodeInterpreter类中添加以下公共函数,用于设置G代码和执行G代码:
```c++
public:
void setGCode(const QString& gCode); // 设置G代码
void execute(); // 执行G代码
```
7. 实现setGCode函数,用于设置G代码:
```c++
void GCodeInterpreter::setGCode(const QString& gCode)
{
m_gCode = gCode.trimmed();
m_lineNumber = 1;
m_variables.clear();
setVariableValue("X", 0.0);
setVariableValue("Y", 0.0);
setVariableValue("Z", 0.0);
}
```
8. 实现execute函数,用于执行G代码:
```c++
void GCodeInterpreter::execute()
{
while (m_lineNumber <= m_gCode.split('\n').count()) {
executeCurrentLine();
}
}
```
9. 在QT项目中的主窗口中添加一个文本框和一个按钮,用于输入G代码和执行G代码。
10. 在主窗口的构造函数中连接按钮的clicked信号到一个槽函数,该槽函数中创建一个GCodeInterpreter对象并调用setGCode和execute函数,将文本框中的G代码作为参数传递给GCodeInterpreter对象。
```c++
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
// ...
connect(ui->executeButton, &QPushButton::clicked, this, [this]() {
GCodeInterpreter interpreter;
interpreter.setGCode(ui->gCodeTextEdit->toPlainText());
interpreter.execute();
});
}
```
11. 运行QT项目,输入一些G代码并点击执行按钮,查看输出结果。
注意:这只是一个简单的G代码解释器的实现,实际中可能需要添加更多的G代码命令和参数解析。
阅读全文
相关推荐
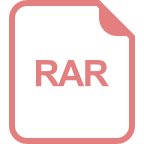
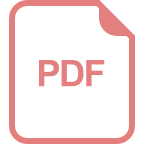
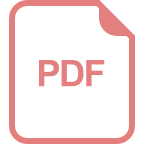















