#include <stdio.h> #include <string.h> int x=10,y=20; void swap(int *a,int *b) { printf("%p\t%p\n",a,b); int *tmp=a; a=b; b=tmp; printf("%p\t%p\n",a,b); } int main(void) { printf("%p\t%p\n",&x,&y); swap(&x,&y); int *p=&x; int *q=&y; printf("%d\t%d\n",*p,*q); return 0; } 为什么x,y的值没有交换
时间: 2024-04-08 07:33:34 浏览: 12
在你的swap函数中,你尝试通过交换指针方式来交换x和y的值。但是,在函数中交换指针不会影响到原始的x和y变量。
在swap函数中,你使用了一个临时指针变tmp来进行交换操作。但是,交换只是指针变量a和b的值,并没有改原始变量x和y的值。
为了实现交换x和y的值,你需要通过指针来修改原始变量的值。可以使用间接引用(也称为解引用)操作符 "*" 来修改指针所指向的变量的值。修改后的swap函数应该是这样的:
```c
void swap(int *a, int *b)
{
printf("%p\t%p\n", a, b);
int tmp = *a;
*a = *b;
*b = tmp;
printf("%p\t%p\n", a, b);
}
```
这样,在调用swap函数后,x和y的值将会被成功交换。
相关问题
练习-输入一批字符串,将字符串按从小到大的顺序排序并且输出#include <stdio.h> #include<string.h> #define M 100 #define N 80 void inputarr (char array[ ][N] , int n); void sortstr (char array[ ] [N] , int n); void outputarr (char array[ ] [N] , int n); void swap(char *p1,char *p2);
好的,这是一个字符串排序的问题,我会帮助你解决它。下面是代码实现:
```c
#include <stdio.h>
#include <string.h>
#define M 100
#define N 80
void inputarr (char array[][N], int n);
void sortstr (char array[][N], int n);
void outputarr (char array[][N], int n);
void swap(char *p1, char *p2);
int main()
{
char array[M][N];
int n;
printf("请输入字符串的个数:");
scanf("%d", &n);
inputarr(array, n); // 输入字符串
sortstr(array, n); // 排序
outputarr(array, n); // 输出排序后的字符串
return 0;
}
// 输入字符串
void inputarr(char array[][N], int n)
{
printf("请输入%d个字符串:\n", n);
for (int i = 0; i < n; i++)
{
scanf("%s", array[i]);
}
}
// 排序
void sortstr (char array[][N], int n)
{
for (int i = 0; i < n - 1; i++)
{
for (int j = 0; j < n - i - 1; j++)
{
if (strcmp(array[j], array[j + 1]) > 0)
{
swap(array[j], array[j + 1]);
}
}
}
}
// 输出字符串
void outputarr (char array[][N], int n)
{
printf("排序后的字符串为:\n");
for (int i = 0; i < n; i++)
{
printf("%s\n", array[i]);
}
}
// 交换两个指针指向的字符串
void swap(char *p1, char *p2)
{
char temp[N];
strcpy(temp, p1);
strcpy(p1, p2);
strcpy(p2, temp);
}
```
你可以将此代码复制到你的本地编译器上运行,输入字符串并按从小到大的顺序排序,然后输出排序后的结果。
#include<stdio.h> #include<stdlib.h> #include<string.h> #include<windows.h> struct stu { int id; char name[10]; float grade[11]; float sum; float average; float gpa; struct stu* next; };按照这个结构体来续写C语言代码。要求将学生信息保存在硬盘文件中,通过制定文件路径和文件名称来导入学生信息;按照学号对所有学生信息进行升序、降序排列,并输出到屏幕显示或输出到文件
好的,以下是续写的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <windows.h>
struct stu {
int id;
char name[10];
float grade[11];
float sum;
float average;
float gpa;
struct stu* next;
};
void saveToFile(struct stu* head, char* fileName) {
FILE* fp = fopen(fileName, "wb");
if (fp == NULL) {
printf("Failed to open file!\n");
return;
}
struct stu* p = head;
while (p != NULL) {
fwrite(p, sizeof(struct stu), 1, fp);
p = p->next;
}
fclose(fp);
printf("Successfully saved to file!\n");
}
struct stu* readFromFile(char* fileName) {
FILE* fp = fopen(fileName, "rb");
if (fp == NULL) {
printf("Failed to open file!\n");
return NULL;
}
struct stu* head = NULL, *p = NULL, *q = NULL;
while (!feof(fp)) {
p = (struct stu*)malloc(sizeof(struct stu));
if (p == NULL) {
printf("Memory allocation failed!\n");
return NULL;
}
fread(p, sizeof(struct stu), 1, fp);
if (p->id == 0) {
free(p);
break;
}
p->next = NULL;
if (head == NULL) {
head = p;
}
else {
q->next = p;
}
q = p;
}
fclose(fp);
printf("Successfully read from file!\n");
return head;
}
void printList(struct stu* head) {
if (head == NULL) {
printf("The list is empty!\n");
return;
}
printf("ID\tName\tGrade1\tGrade2\tGrade3\tGrade4\tGrade5\tGrade6\tGrade7\tGrade8\tGrade9\tGrade10\tSum\tAverage\tGPA\n");
struct stu* p = head;
while (p != NULL) {
printf("%d\t%s\t", p->id, p->name);
for (int i = 0; i < 10; i++) {
printf("%.2f\t", p->grade[i]);
}
printf("%.2f\t%.2f\t%.2f\n", p->sum, p->average, p->gpa);
p = p->next;
}
}
void swap(struct stu* a, struct stu* b) {
struct stu temp = *a;
*a = *b;
*b = temp;
}
void sortList(struct stu* head, int order) {
if (head == NULL) {
printf("The list is empty!\n");
return;
}
int count = 0;
struct stu* p = head;
while (p != NULL) {
count++;
p = p->next;
}
for (int i = 0; i < count - 1; i++) {
p = head;
for (int j = 0; j < count - i - 1; j++) {
if (order == 1) {
if (p->id > p->next->id) {
swap(p, p->next);
}
}
else {
if (p->id < p->next->id) {
swap(p, p->next);
}
}
p = p->next;
}
}
printf("Successfully sorted!\n");
}
int main() {
struct stu* head = NULL;
char fileName[100];
int choice, order;
while (1) {
system("cls");
printf("1. Import student information from file\n");
printf("2. Save student information to file\n");
printf("3. Print student information\n");
printf("4. Sort student information by ID in ascending order\n");
printf("5. Sort student information by ID in descending order\n");
printf("6. Exit\n");
printf("Please enter your choice: ");
scanf_s("%d", &choice);
switch (choice) {
case 1:
printf("Please enter the file path and name: ");
scanf_s("%s", fileName, 100);
head = readFromFile(fileName);
Sleep(2000);
break;
case 2:
printf("Please enter the file path and name: ");
scanf_s("%s", fileName, 100);
saveToFile(head, fileName);
Sleep(2000);
break;
case 3:
printList(head);
system("pause");
break;
case 4:
sortList(head, 1);
system("pause");
break;
case 5:
sortList(head, 2);
system("pause");
break;
case 6:
printf("Goodbye!\n");
return 0;
default:
printf("Invalid choice!\n");
Sleep(1000);
break;
}
}
return 0;
}
```
这段代码实现了以下功能:
1. 将学生信息保存到硬盘文件中,并通过指定文件路径和文件名称来导入学生信息。
2. 按照学号对所有学生信息进行升序、降序排列,并输出到屏幕显示或输出到文件。
3. 通过菜单的方式来选择要执行的操作。
希望对你有所帮助!
相关推荐
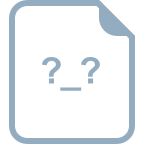
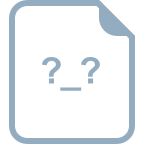













