c语言生成怎么生成两个图形窗口
时间: 2023-12-05 10:04:36 浏览: 78
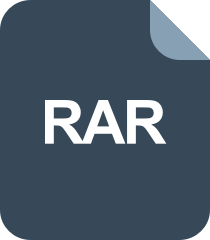
C语言的窗口图形编程
在 C 语言中,可以使用不同的图形库来生成窗口。其中比较常用的是 Windows API 和 OpenGL。
使用 Windows API,可以使用 CreateWindow 函数创建一个新的窗口,例如:
```c
#include <windows.h>
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
// Register the window class.
const char CLASS_NAME[] = "My Window Class";
WNDCLASS wc = { };
wc.lpfnWndProc = WindowProc;
wc.hInstance = hInstance;
wc.lpszClassName = CLASS_NAME;
RegisterClass(&wc);
// Create the window.
HWND hwnd = CreateWindowEx(
0, // Optional window styles.
CLASS_NAME, // Window class
"Window 1", // Window title
WS_OVERLAPPEDWINDOW, // Window style
// Size and position
CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT,
NULL, // Parent window
NULL, // Menu
hInstance, // Instance handle
NULL // Additional application data
);
// Show the window
ShowWindow(hwnd, nCmdShow);
// Create a second window.
HWND hwnd2 = CreateWindowEx(
0, // Optional window styles.
CLASS_NAME, // Window class
"Window 2", // Window title
WS_OVERLAPPEDWINDOW, // Window style
// Size and position
CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT, CW_USEDEFAULT,
NULL, // Parent window
NULL, // Menu
hInstance, // Instance handle
NULL // Additional application data
);
// Show the second window
ShowWindow(hwnd2, nCmdShow);
// Run the message loop.
MSG msg = { };
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return 0;
}
LRESULT CALLBACK WindowProc(HWND hwnd, UINT uMsg, WPARAM wParam, LPARAM lParam)
{
switch (uMsg)
{
case WM_DESTROY:
PostQuitMessage(0);
return 0;
default:
return DefWindowProc(hwnd, uMsg, wParam, lParam);
}
}
```
这个程序会创建两个窗口,分别显示 "Window 1" 和 "Window 2"。
使用 OpenGL,可以使用 glut 库,例如:
```c
#include <GL/glut.h>
void display1()
{
// Set the clear color to black
glClearColor(0.0f, 0.0f, 0.0f, 1.0f);
// Clear the screen
glClear(GL_COLOR_BUFFER_BIT);
// Draw a red triangle
glBegin(GL_TRIANGLES);
glColor3f(1.0f, 0.0f, 0.0f);
glVertex2f(-0.5f, -0.5f);
glVertex2f( 0.5f, -0.5f);
glVertex2f( 0.0f, 0.5f);
glEnd();
// Swap the buffers
glutSwapBuffers();
}
void display2()
{
// Set the clear color to blue
glClearColor(0.0f, 0.0f, 1.0f, 1.0f);
// Clear the screen
glClear(GL_COLOR_BUFFER_BIT);
// Draw a green rectangle
glBegin(GL_QUADS);
glColor3f(0.0f, 1.0f, 0.0f);
glVertex2f(-0.5f, -0.5f);
glVertex2f( 0.5f, -0.5f);
glVertex2f( 0.5f, 0.5f);
glVertex2f(-0.5f, 0.5f);
glEnd();
// Swap the buffers
glutSwapBuffers();
}
int main(int argc, char** argv)
{
glutInit(&argc, argv);
// Create the first window
glutInitWindowSize(400, 300);
glutInitWindowPosition(100, 100);
glutCreateWindow("Window 1");
// Set the display function for the first window
glutDisplayFunc(display1);
// Create the second window
glutInitWindowSize(400, 300);
glutInitWindowPosition(500, 100);
glutCreateWindow("Window 2");
// Set the display function for the second window
glutDisplayFunc(display2);
// Start the main loop
glutMainLoop();
return 0;
}
```
这个程序会创建两个窗口,分别显示一个红色的三角形和一个绿色的矩形。
阅读全文
相关推荐
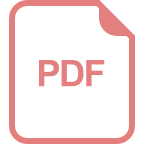
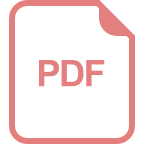
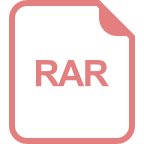
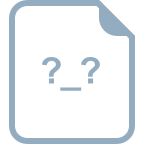
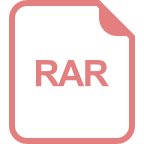
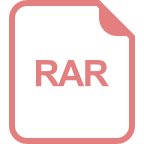
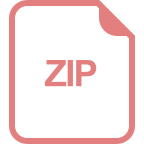
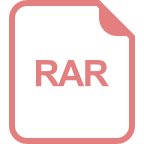
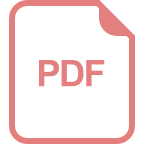
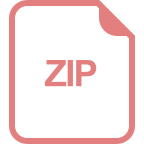
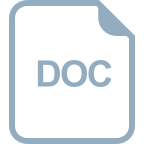
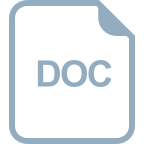
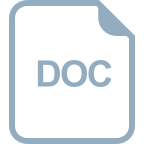
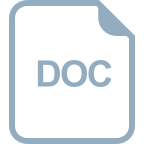
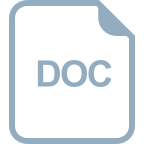
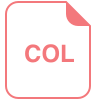