用 java中的 Teigha 实现读取dwg文件中所有信息
时间: 2024-02-26 09:58:15 浏览: 349
要读取DWG文件中的所有信息,可以使用Teigha的一些API,如OdDbBlockTable、OdDbEntity、OdDbLayerTable等。以下是一个示例代码,可以读取DWG文件中的所有块、实体、图层等信息:
```java
import com.opendesign.oddb.*;
import com.opendesign.oddb.entity.*;
public class ReadDWG {
public static void main(String[] args) {
try {
// 创建一个Teigha数据库对象
OdDbDatabase db = new OdDbDatabase(false, true);
// 打开DWG文件
db.readDwgFile("path/to/your/file.dwg");
// 输出DWG文件的版本信息
System.out.println("DWG file version: " + db.getVersion());
// 读取所有块
OdDbBlockTable blockTable = db.getBlockTable();
for (OdDbBlockTableRecord block : blockTable) {
System.out.println("Block name: " + block.getName());
// 读取块中的实体
OdDbBlockTableRecordIterator iterator = block.iterator();
while (iterator.hasNext()) {
OdDbEntity entity = iterator.next();
System.out.println("Entity type: " + entity.getClass().getSimpleName());
}
}
// 读取所有实体
OdDbBlockTableRecord modelSpace = db.getModelSpace();
OdDbBlockTableRecordIterator iterator = modelSpace.iterator();
while (iterator.hasNext()) {
OdDbEntity entity = iterator.next();
System.out.println("Entity type: " + entity.getClass().getSimpleName());
}
// 读取所有图层
OdDbLayerTable layerTable = db.getLayerTable();
for (OdDbLayerTableRecord layer : layerTable) {
System.out.println("Layer name: " + layer.getName());
// 读取图层中的实体
OdDbBlockTableRecord layerRecord = layer.getRecord();
iterator = layerRecord.iterator();
while (iterator.hasNext()) {
OdDbEntity entity = iterator.next();
System.out.println("Entity type: " + entity.getClass().getSimpleName());
}
}
// 关闭数据库
db.close();
} catch (OdDbException e) {
e.printStackTrace();
}
}
}
```
需要注意的是,在读取DWG文件中的实体时,需要根据实体的类型进行转换,如OdDbLine、OdDbCircle、OdDbText等。可以通过实体的类型名称来进行判断和转换。具体使用方法可以参考Teigha的官方文档。
阅读全文
相关推荐





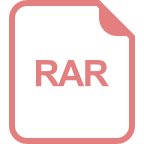









