帮我写一套 Pygame 的 GUI 控件
时间: 2024-02-21 15:00:10 浏览: 190
好的,我可以为你提供一个简单的 Pygame GUI 控件库。这个控件库包括以下控件:
- Label:用于显示文本。
- Button:用于触发事件。
- InputBox:用于接收用户输入。
- MessageBox:用于显示消息。
请在安装 Pygame 包后使用以下代码创建 Pygame GUI 控件库:
```python
import pygame
# 初始化 Pygame
pygame.init()
# 定义颜色
white = (255, 255, 255)
black = (0, 0, 0)
gray = (128, 128, 128)
# 创建 Label 类
class Label:
def __init__(self, text, font_size, x, y, color=black):
self.font = pygame.font.Font(None, font_size)
self.text = self.font.render(text, True, color)
self.rect = self.text.get_rect()
self.rect.x = x
self.rect.y = y
def draw(self, screen):
screen.blit(self.text, self.rect)
# 创建 Button 类
class Button:
def __init__(self, text, font_size, x, y, color=gray, hover_color=white, callback=None):
self.font = pygame.font.Font(None, font_size)
self.text = self.font.render(text, True, color)
self.rect = self.text.get_rect()
self.rect.x = x
self.rect.y = y
self.color = color
self.hover_color = hover_color
self.callback = callback
def draw(self, screen):
mouse_pos = pygame.mouse.get_pos()
if self.rect.collidepoint(mouse_pos):
pygame.draw.rect(screen, self.hover_color, self.rect)
else:
pygame.draw.rect(screen, self.color, self.rect)
screen.blit(self.text, self.rect)
def handle_event(self, event):
if event.type == pygame.MOUSEBUTTONDOWN and event.button == 1:
mouse_pos = pygame.mouse.get_pos()
if self.rect.collidepoint(mouse_pos):
if self.callback:
self.callback()
# 创建 InputBox 类
class InputBox:
def __init__(self, font_size, x, y, width, height, color=white, active_color=gray):
self.font = pygame.font.Font(None, font_size)
self.text = ""
self.rect = pygame.Rect(x, y, width, height)
self.color = color
self.active_color = active_color
self.active = False
def draw(self, screen):
pygame.draw.rect(screen, self.active_color if self.active else self.color, self.rect, 2)
text_surface = self.font.render(self.text, True, black)
screen.blit(text_surface, (self.rect.x + 5, self.rect.y + 5))
def handle_event(self, event):
if event.type == pygame.MOUSEBUTTONDOWN and event.button == 1:
self.active = self.rect.collidepoint(event.pos)
if event.type == pygame.KEYDOWN:
if self.active:
if event.key == pygame.K_BACKSPACE:
self.text = self.text[:-1]
else:
self.text += event.unicode
# 创建 MessageBox 类
class MessageBox:
def __init__(self, text, font_size, x, y, width, height, color=white):
self.font = pygame.font.Font(None, font_size)
self.text = text
self.color = color
self.rect = pygame.Rect(x, y, width, height)
def draw(self, screen):
pygame.draw.rect(screen, self.color, self.rect)
text_surface = self.font.render(self.text, True, black)
screen.blit(text_surface, (self.rect.x + 5, self.rect.y + 5))
# 创建 GUI 类
class GUI:
def __init__(self, width, height, title):
self.width = width
self.height = height
self.title = title
self.screen = pygame.display.set_mode((self.width, self.height))
pygame.display.set_caption(self.title)
self.clock = pygame.time.Clock()
self.widgets = []
def add_widget(self, widget):
self.widgets.append(widget)
def run(self):
done = False
while not done:
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
for widget in self.widgets:
widget.handle_event(event)
self.screen.fill(white)
for widget in self.widgets:
widget.draw(self.screen)
pygame.display.flip()
self.clock.tick(60)
pygame.quit()
```
这个控件库包括 Label、Button、InputBox、MessageBox 四个控件,你可以根据需要对这些控件进行修改和扩展。控件库的使用方法如下所示:
```python
# 创建 GUI 对象
gui = GUI(800, 600, "Pygame GUI")
# 创建 Label 对象
label = Label("Hello, Pygame GUI!", 32, 200, 200)
# 创建 Button 对象
def on_click():
print("Button clicked")
button = Button("Click me", 24, 200, 300, callback=on_click)
# 创建 InputBox 对象
input_box = InputBox(24, 200, 400, 200, 30)
# 创建 MessageBox 对象
message_box = MessageBox("This is a message box", 24, 200, 500, 200, 30)
# 添加控件到 GUI 中
gui.add_widget(label)
gui.add_widget(button)
gui.add_widget(input_box)
gui.add_widget(message_box)
# 运行 GUI
gui.run()
```
在这个例子中,我们创建了一个 GUI 对象,然后创建了一个 Label、一个 Button、一个 InputBox 和一个 MessageBox 对象,并将这些控件添加到 GUI 中。最后,我们调用 run() 方法来运行 GUI。在 GUI 运行过程中,我们可以通过回调函数来响应 Button 的点击事件,也可以通过 InputBox 来接收用户输入。
阅读全文
相关推荐
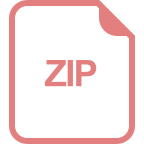
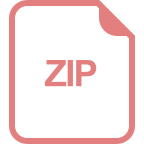
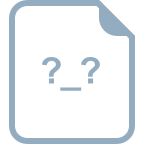
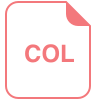
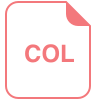
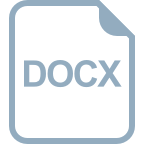
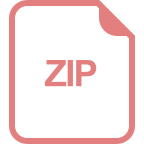
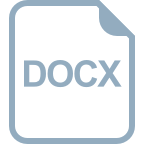
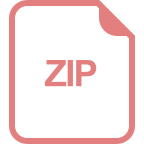
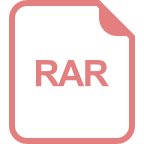
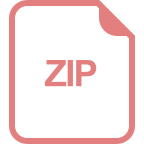
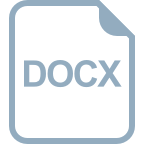
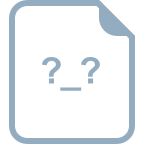
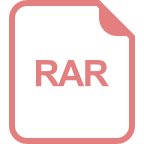