用Qt widget 实现炫酷的轮播图, 要求可以显示多张图片, 每次只显示5张立体堆叠的具体代码
时间: 2024-04-21 12:28:48 浏览: 192
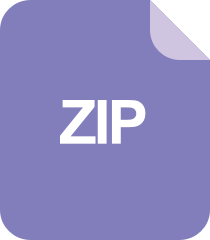
glwidget_QT_用QT的opengl显示图片_

好的,以下是一个简单的Qt widget实现炫酷的轮播图,每次只显示5张立体堆叠的具体代码:
```python
from PyQt5.QtCore import QTimer, QPropertyAnimation, QRect, Qt, pyqtProperty, pyqtSignal
from PyQt5.QtGui import QPainter, QPixmap
from PyQt5.QtWidgets import QWidget, QHBoxLayout
class CarouselWidget(QWidget):
def __init__(self, parent=None):
super().__init__(parent)
# 图片资源
self.images = []
# 布局管理器
self.layout = QHBoxLayout(self)
self.layout.setContentsMargins(0, 0, 0, 0)
self.setLayout(self.layout)
# 定时器
self.timer = QTimer(self)
self.timer.timeout.connect(self.show_next_images)
self.timer.start(3000)
# 动画
self.animation = QPropertyAnimation(self, b"offset")
self.animation.setDuration(500)
self.animation.setEasingCurve(Qt.OutQuad)
# 偏移量
self._offset = 0
# 偏移量属性
@pyqtProperty(int)
def offset(self):
return self._offset
@offset.setter
def offset(self, value):
self._offset = value
self.update()
# 绘制立体效果
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
for i in range(5):
index = i - 2 + self._offset
if index < 0:
index += len(self.images)
if index >= len(self.images):
index -= len(self.images)
pixmap = self.images[index]
rect = QRect(i * self.width() / 5, 0, self.width() / 5, self.height())
if i == 2:
painter.drawPixmap(rect, pixmap)
elif i < 2:
painter.setOpacity(1 - 0.2 * i)
painter.drawPixmap(rect.adjusted(0, 0, -self.width() / 10, 0), pixmap)
else:
painter.setOpacity(1 - 0.2 * (4 - i))
painter.drawPixmap(rect.adjusted(self.width() / 10, 0, 0, 0), pixmap)
# 动态调整图片大小和布局
def resizeEvent(self, event):
super().resizeEvent(event)
for i in range(self.layout.count()):
widget = self.layout.itemAt(i).widget()
widget.setFixedSize(self.width() / 5, self.height())
self.layout.setSpacing(-self.width() / 10)
# 手势滑动效果
def mousePressEvent(self, event):
self.animation.stop()
self._start_pos = event.pos().x()
def mouseReleaseEvent(self, event):
delta = event.pos().x() - self._start_pos
if delta > self.width() / 10:
self.show_prev_images()
elif delta < -self.width() / 10:
self.show_next_images()
else:
self.animation.setStartValue(self._offset)
self.animation.setEndValue(self._offset)
self.animation.start()
# 显示上五张图片
def show_prev_images(self):
self.animation.setStartValue(self._offset)
self.animation.setEndValue(self._offset - 5)
self.animation.start()
# 显示下五张图片
def show_next_images(self):
self.animation.setStartValue(self._offset)
self.animation.setEndValue(self._offset + 5)
self.animation.start()
# 设置要显示的图片
def set_images(self, images):
self.images = images
for pixmap in self.images:
widget = QWidget(self)
widget.setFixedSize(self.width() / 5, self.height())
layout = QHBoxLayout(widget)
layout.setContentsMargins(0, 0, 0, 0)
label = QLabel(widget)
label.setPixmap(pixmap.scaled(widget.size(), Qt.KeepAspectRatio, Qt.SmoothTransformation))
layout.addWidget(label)
widget.setLayout(layout)
self.layout.addWidget(widget)
```
在这个实现中,我们使用了QHBoxLayout来管理图片的布局,使用QTimer来定时切换图片。我们还使用了QPropertyAnimation和手势滑动效果来实现图片的平移动画效果。在绘制立体效果时,我们使用了QPainter来绘制出不同位置的图片,并设置了不同的透明度,以实现立体堆叠的效果。
阅读全文
相关推荐
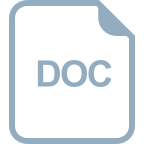
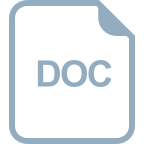

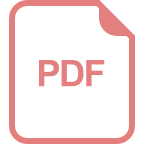
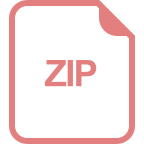
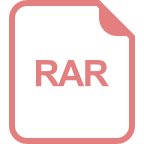
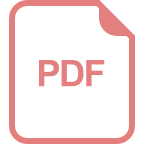
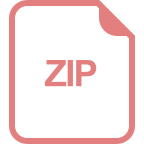
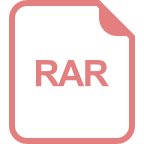
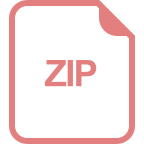
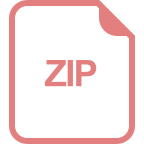
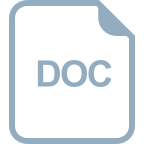
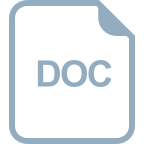

