使用poi读取excel数据读取图片
时间: 2023-11-22 19:08:13 浏览: 156
可以使用Apache POI库来读取Excel数据并提取图片。以下是一个简单的示例代码:
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
import org.apache.poi.util.IOUtils;
import org.apache.poi.xssf.usermodel.XSSFClientAnchor;
import org.apache.poi.xssf.usermodel.XSSFPicture;
import org.apache.poi.xssf.usermodel.XSSFPictureData;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ReadExcelWithImage {
public static void main(String[] args) throws IOException {
String filePath = "path/to/excel/file.xlsx";
InputStream inputStream = new FileInputStream(filePath);
Workbook workbook = WorkbookFactory.create(inputStream);
int sheetCount = workbook.getNumberOfSheets();
for (int i = 0; i < sheetCount; i++) {
Sheet sheet = workbook.getSheetAt(i);
if (sheet instanceof XSSFSheet) {
XSSFSheet xssfSheet = (XSSFSheet) sheet;
for (Row row : xssfSheet) {
for (Cell cell : row) {
switch (cell.getCellType()) {
case STRING:
System.out.print(cell.getStringCellValue() + "\t");
break;
case NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
case BOOLEAN:
System.out.print(cell.getBooleanCellValue() + "\t");
break;
case FORMULA:
System.out.print(cell.getCellFormula() + "\t");
break;
default:
System.out.print(" " + "\t");
}
if (cell.getCellType() == Cell.CELL_TYPE_FORMULA) {
switch (cell.getCachedFormulaResultType()) {
case Cell.CELL_TYPE_NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
case Cell.CELL_TYPE_STRING:
System.out.print(cell.getStringCellValue() + "\t");
break;
}
}
if (cell.getCellType() == Cell.CELL_TYPE_BLANK) {
System.out.print(" " + "\t");
}
if (cell.getCellType() == Cell.CELL_TYPE_ERROR) {
System.out.print(cell.getErrorCellValue() + "\t");
}
if (cell.getCellType() == Cell.CELL_TYPE_BOOLEAN) {
System.out.print(cell.getBooleanCellValue() + "\t");
}
if (cell.getCellType() == Cell.CELL_TYPE_NUMERIC) {
System.out.print(cell.getNumericCellValue() + "\t");
}
if (cell.getCellType() == Cell.CELL_TYPE_STRING) {
System.out.print(cell.getStringCellValue() + "\t");
}
if (cell.getCellType() == Cell.CELL_TYPE_BLANK) {
System.out.print("" + "\t");
}
if (cell.getCellType() == Cell.CELL_TYPE_FORMULA
&& cell.getCachedFormulaResultType() == Cell.CELL_TYPE_NUMERIC) {
System.out.print(cell.getNumericCellValue() + "\t");
}
if (cell.getCellType() == Cell.CELL_TYPE_FORMULA
&& cell.getCachedFormulaResultType() == Cell.CELL_TYPE_STRING) {
System.out.print(cell.getStringCellValue() + "\t");
}
// get image from cell
if (cell.getCellType() == Cell.CELL_TYPE_NUMERIC) {
if (xssfSheet.getDrawingPatriarch() != null) {
for (XSSFPicture picture : xssfSheet.getDrawingPatriarch().getShapes().getPictures()) {
XSSFClientAnchor anchor = picture.getClientAnchor();
if (anchor.getRow1() == row.getRowNum() && anchor.getCol1() == cell.getColumnIndex()) {
XSSFPictureData pictureData = picture.getPictureData();
byte[] bytes = pictureData.getData();
String extension = pictureData.suggestFileExtension();
String fileName = "image" + row.getRowNum() + "_" + cell.getColumnIndex() + "." + extension;
IOUtils.write(bytes, new FileOutputStream(fileName));
}
}
}
}
}
System.out.println("");
}
}
}
}
}
```
在这个例子中,我们遍历每个单元格,并根据其类型打印出其内容。如果单元格包含图片,我们使用Apache POI的XSSFDrawing对象来提取图像并将其保存到文件中。
请注意,上面的代码仅适用于XLSX格式的Excel文件。如果您的Excel文件是旧的XLS格式,您需要使用HSSFWorkbook和HSSFPicture类来读取图像。
相关推荐
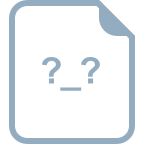
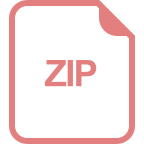

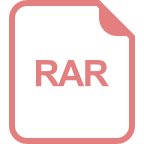
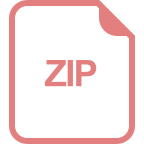
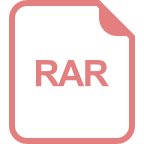
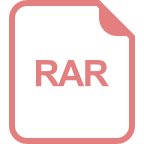
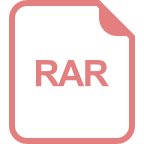
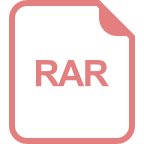
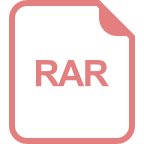
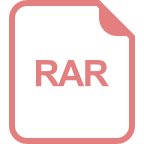
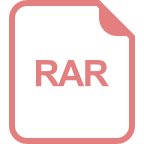
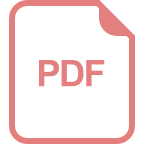
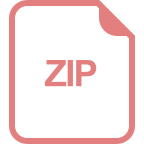
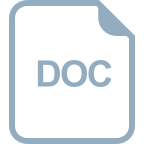
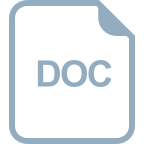

