ffmpeg qsv编解码
时间: 2024-01-22 20:16:32 浏览: 225
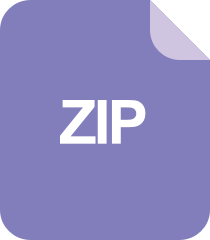
ffmpeg编解码
ffmpeg是一个开源的音视频处理工具,可以用于音视频的编解码、转换、处理等操作。QSV(Quick Sync Video)是Intel提供的硬件加速技术,可以在支持的Intel处理器上进行视频编解码操作。
要使用ffmpeg进行QSV编解码,需要先安装ffmpeg,并确保系统的硬件支持QSV。然后可以使用ffmpeg命令行或者在代码中调用ffmpeg库进行编解码操作。
在代码中使用QSV进行编解码的示例代码如下:
```c++
#include <stdio.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
int main(int argc, char* argv[]) {
AVFormatContext* fmt_ctx = NULL;
AVCodecContext* dec_ctx = NULL;
AVCodecContext* enc_ctx = NULL;
AVPacket pkt;
AVFrame* frame = NULL;
AVFrame* enc_frame = NULL;
AVCodec* dec_codec = NULL;
AVCodec* enc_codec = NULL;
int ret;
// 初始化ffmpeg
av_register_all();
avcodec_register_all();
// 打开输入文件
ret = avformat_open_input(&fmt_ctx, "input.mp4", NULL, NULL);
if (ret < 0) {
printf("Failed to open input file\n");
return ret;
}
// 查找视频流
ret = avformat_find_stream_info(fmt_ctx, NULL);
if (ret < 0) {
printf("Failed to find stream info\n");
return ret;
}
// 查找视频解码器
dec_codec = avcodec_find_decoder_by_name("h264_qsv");
if (!dec_codec) {
printf("Failed to find decoder\n");
return AVERROR_DECODER_NOT_FOUND;
}
// 创建解码器上下文
dec_ctx = avcodec_alloc_context3(dec_codec);
if (!dec_ctx) {
printf("Failed to allocate decoder context\n");
return AVERROR(ENOMEM);
}
// 打开解码器
ret = avcodec_open2(dec_ctx, dec_codec, NULL);
if (ret < 0) {
printf("Failed to open decoder\n");
return ret;
}
// 初始化编码器
enc_codec = avcodec_find_encoder_by_name("h264_qsv");
if (!enc_codec) {
printf("Failed to find encoder\n");
return AVERROR_ENCODER_NOT_FOUND;
}
// 创建编码器上下文
enc_ctx = avcodec_alloc_context3(enc_codec);
if (!enc_ctx) {
printf("Failed to allocate encoder context\n");
return AVERROR(ENOMEM);
}
// 设置编码器参数
enc_ctx->width = dec_ctx->width;
enc_ctx->height = dec_ctx->height;
enc_ctx->pix_fmt = AV_PIX_FMT_NV12;
enc_ctx->time_base = dec_ctx->time_base;
// 打开编码器
ret = avcodec_open2(enc_ctx, enc_codec, NULL);
if (ret < 0) {
printf("Failed to open encoder\n");
return ret;
}
// 分配帧内存
frame = av_frame_alloc();
enc_frame = av_frame_alloc();
if (!frame || !enc_frame) {
printf("Failed to allocate frame\n");
return AVERROR(ENOMEM);
}
// 循环读取视频帧并进行编解码
while (av_read_frame(fmt_ctx, &pkt) >= 0) {
if (pkt.stream_index == video_stream_index) {
// 解码视频帧
ret = avcodec_send_packet(dec_ctx, &pkt);
if (ret < 0) {
printf("Failed to send packet to decoder\n");
break;
}
while (ret >= 0) {
ret = avcodec_receive_frame(dec_ctx, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
printf("Failed to receive frame from decoder\n");
break;
}
// 编码视频帧
ret = avcodec_send_frame(enc_ctx, frame);
if (ret < 0) {
printf("Failed to send frame to encoder\n");
break;
}
while (ret >= 0) {
ret = avcodec_receive_packet(enc_ctx, &pkt);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
printf("Failed to receive packet from encoder\n");
break;
}
// 处理编码后的数据
// ...
av_packet_unref(&pkt);
}
av_frame_unref(frame);
}
}
av_packet_unref(&pkt);
}
// 清理资源
av_frame_free(&frame);
av_frame_free(&enc_frame);
avcodec_free_context(&dec_ctx);
avcodec_free_context(&enc_ctx);
avformat_close_input(&fmt_ctx);
return 0;
}
```
这段代码演示了使用ffmpeg进行QSV编解码的基本流程,包括打开输入文件、查找解码器、打开解码器、初始化编码器、打开编码器、循环读取视频帧并进行编解码等操作。
阅读全文
相关推荐
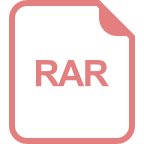

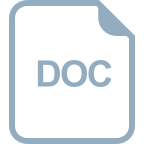


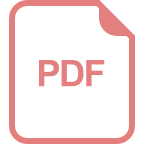
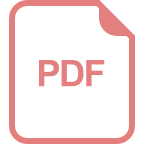
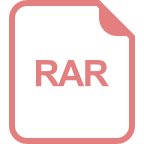
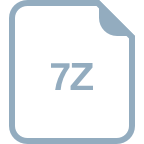





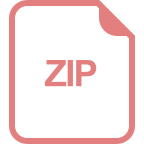
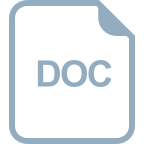
