from future import annotations from song import Song
时间: 2024-09-22 15:07:29 浏览: 62
`from future import annotations` 这一行导入语句在Python编程中用于引入Python 3.7及更高版本中的类型注解功能。类型注解允许程序员在函数、变量等声明中指定它们的数据类型,虽然Python本身并不强制执行这些注解,但它可以帮助开发者更好地理解和文档化代码,并在一些工具中提供类型检查。
`from song import Song` 则是导入了一个名为 `Song` 的模块或类。`Song` 可能是一个包含歌曲类的文件,这个类可能有属性如歌曲名、歌手、歌词等,以及相关的操作方法。通过这行代码,你可以直接在当前作用域下使用 `Song` 类及其成员。
举个例子,假设`Song.py` 文件中有这样的定义:
```python
class Song:
def __init__(self, title: str, artist: str):
self.title = title
self.artist = artist
def sing(self) -> None:
print(f"{self.artist} is singing '{self.title}'")
```
在导入后,你就可以创建 `Song` 实例并调用其方法:
```python
my_song = Song("Yesterday", "The Beatles")
my_song.sing() # 输出: The Beatles is singing 'Yesterday'
```
相关问题
from __future__ import annotations SyntaxError: future feature annotations is not defined
这个错误通常是因为您的Python版本不支持使用__future__模块中的annotations特性导致的。
在Python 3.7之前的版本中,annotations特性是作为实验性特性引入的,需要使用__future__模块中的annotations来启用。但是在Python 3.7及以后的版本中,annotations成为了官方特性,不再需要使用__future__模块来启用。
因此,您可以尝试升级您的Python版本,或者在Python 3.7及以后的版本中不使用__future__模块中的annotations特性。
from __future__ import annotations ^ SyntaxError: future feature annotations is not defined
这个错误通常是由于你使用了Python版本较低导致的,因为 `__future__` 模块是为了引入一些新特性,这些特性在旧版本的Python中并不存在。
解决方法是升级Python版本,或者在代码的开头添加以下代码:
```python
# -*- coding: utf-8 -*-
from __future__ import annotations
```
这样可以确保你的代码可以在旧版本的Python中运行,同时也可以确保代码在新版本的Python中使用新特性。
阅读全文
相关推荐
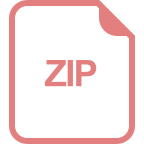
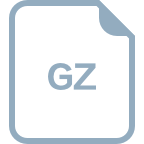
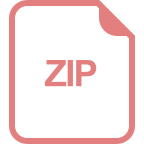











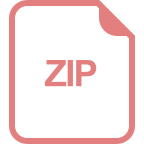