fopen_s的用法
时间: 2024-06-14 16:04:02 浏览: 20
fopen_s是C语言中的一个安全版本的文件打开函数,用于打开文件并返回文件指针。它的用法如下所示:
```c
#include <stdio.h>
int fopen_s(FILE** pFile, const char* filename, const char* mode);
```
其中,pFile是一个指向FILE指针的指针,用于接收打开的文件指针;filename是要打开的文件名;mode是打开文件的模式。
下面是一个使用fopen_s函数的示例代码:
```c
#include <stdio.h>
int main() {
FILE* fp = NULL;
errno_t err;
err = fopen_s(&fp, "D:\\users.txt", "r");
if (err != 0) {
perror("failed to open");
return -1;
}
// 文件操作
fclose(fp);
return 0;
}
```
在这个示例中,我们首先定义了一个FILE指针fp,并初始化为NULL。然后使用fopen_s函数打开名为"D:\\users.txt"的文件,以只读模式打开。如果打开文件失败,会输出错误信息并返回-1。如果打开文件成功,我们可以在文件操作部分进行读取或写入操作。最后,使用fclose函数关闭文件。
相关推荐
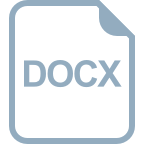
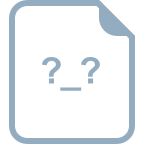
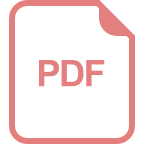
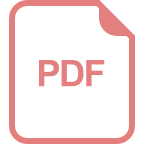
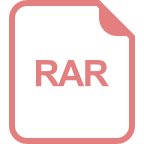
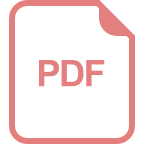
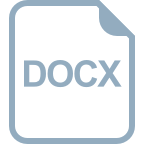
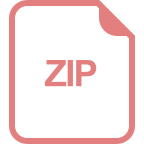
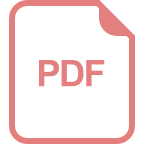
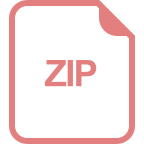
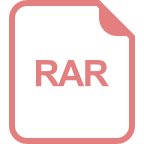
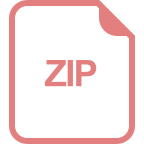