C++ 查找字符串里的所有不同特定字符,并把他们去除,不改变其他字符的位置
时间: 2024-09-13 07:06:04 浏览: 34
在C++中,你可以通过以下几个步骤查找并移除字符串中的所有特定字符:
1. 定义目标字符:首先确定你要从字符串中移除的特定字符,例如字符数组或`char`类型的变量。
```cpp
const char targetChar = 'a'; // 或者是其他你想移除的字符
```
2. 创建一个新的字符串来存储结果:声明一个新的动态大小的字符串,用于保存原字符串去掉特定字符后的内容。
```cpp
std::string result;
```
3. 遍历原字符串:使用`std::string`的迭代器遍历原字符串,判断每个字符是否为目标字符,如果不是,则添加到新字符串中。
```cpp
for (auto it = originalString.begin(); it != originalString.end(); ++it) {
if (*it != targetChar) {
result += *it; // 这里假设我们使用+=来追加字符到新字符串
}
}
```
4. 结果字符串作为新的字符串返回:遍历结束后,原字符串中的特定字符已经去除,`result`就是你需要的结果。
完整示例:
```cpp
#include <iostream>
#include <string>
std::string removeSpecificChars(const std::string& originalString, char targetChar) {
std::string result;
for (auto it = originalString.begin(); it != originalString.end(); ++it) {
if (*it != targetChar) {
result += *it;
}
}
return result;
}
int main() {
std::string str = "Hello, world!";
char toRemove = 'l';
std::cout << "Original string: " << str << "\n";
std::string newStr = removeSpecificChars(str, toRemove);
std::cout << "After removing '" << toRemove << "': " << newStr << "\n";
return 0;
}
```
运行此代码会输出去除了所有'l'的新字符串。
阅读全文
相关推荐
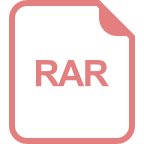
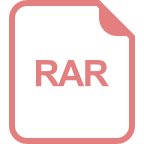
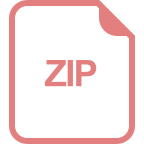












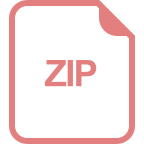
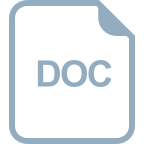
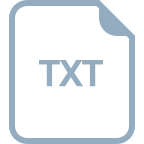
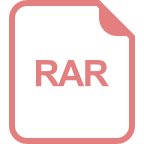