用c#连接数据库写一个简单的购物系统
时间: 2023-10-19 18:05:13 浏览: 41
好的,以下是一个简单的购物系统的 C# 代码示例:
```csharp
using System;
using System.Data.SqlClient;
namespace ShoppingSystem
{
class Program
{
static void Main(string[] args)
{
string connectionString = "Data Source=ServerName;Initial Catalog=DatabaseName;User ID=UserName;Password=Password";
SqlConnection connection = new SqlConnection(connectionString);
connection.Open();
Console.WriteLine("欢迎使用购物系统!");
Console.WriteLine();
while (true)
{
Console.WriteLine("请选择操作:");
Console.WriteLine("1. 查看商品列表");
Console.WriteLine("2. 添加商品到购物车");
Console.WriteLine("3. 查看购物车");
Console.WriteLine("4. 结算");
Console.WriteLine("5. 退出");
Console.WriteLine();
string choice = Console.ReadLine();
if (choice == "1")
{
Console.WriteLine("商品列表:");
Console.WriteLine();
SqlCommand command = new SqlCommand("SELECT * FROM Products", connection);
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
Console.WriteLine("{0} - {1} - ¥{2}", reader["Id"], reader["Name"], reader["Price"]);
}
reader.Close();
Console.WriteLine();
}
else if (choice == "2")
{
Console.WriteLine("请输入要添加的商品编号:");
int productId = int.Parse(Console.ReadLine());
SqlCommand command = new SqlCommand("SELECT * FROM Products WHERE Id = @Id", connection);
command.Parameters.AddWithValue("@Id", productId);
SqlDataReader reader = command.ExecuteReader();
if (reader.Read())
{
Console.WriteLine("已添加商品:{0} - ¥{1}", reader["Name"], reader["Price"]);
reader.Close();
command = new SqlCommand("INSERT INTO ShoppingCart (ProductId) VALUES (@ProductId)", connection);
command.Parameters.AddWithValue("@ProductId", productId);
command.ExecuteNonQuery();
}
else
{
Console.WriteLine("未找到商品!");
reader.Close();
}
Console.WriteLine();
}
else if (choice == "3")
{
Console.WriteLine("购物车中的商品:");
Console.WriteLine();
SqlCommand command = new SqlCommand("SELECT Products.Name, Products.Price FROM ShoppingCart INNER JOIN Products ON ShoppingCart.ProductId = Products.Id", connection);
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
Console.WriteLine("{0} - ¥{1}", reader["Name"], reader["Price"]);
}
reader.Close();
Console.WriteLine();
}
else if (choice == "4")
{
Console.WriteLine("结算:");
SqlCommand command = new SqlCommand("SELECT SUM(Products.Price) AS TotalPrice FROM ShoppingCart INNER JOIN Products ON ShoppingCart.ProductId = Products.Id", connection);
SqlDataReader reader = command.ExecuteReader();
if (reader.Read())
{
Console.WriteLine("总价:¥{0}", reader["TotalPrice"]);
}
reader.Close();
command = new SqlCommand("DELETE FROM ShoppingCart", connection);
command.ExecuteNonQuery();
Console.WriteLine("谢谢惠顾!");
Console.WriteLine();
}
else if (choice == "5")
{
break;
}
else
{
Console.WriteLine("无效的选择!");
Console.WriteLine();
}
}
connection.Close();
}
}
}
```
注意,这个代码示例中使用了 SQL Server 数据库,并且需要将 `ServerName`、`DatabaseName`、`UserName` 和 `Password` 替换成实际的值。同时,还需要在数据库中创建 `Products` 和 `ShoppingCart` 表,具体的表结构如下:
```sql
CREATE TABLE Products (
Id INT PRIMARY KEY,
Name VARCHAR(50),
Price DECIMAL(18, 2)
);
CREATE TABLE ShoppingCart (
Id INT PRIMARY KEY IDENTITY,
ProductId INT FOREIGN KEY REFERENCES Products(Id)
);
```
这个购物系统的逻辑很简单,就是可以查看商品列表、添加商品到购物车、查看购物车和结算。当用户选择结算时,程序会计算购物车中的商品总价,并清空购物车。
相关推荐
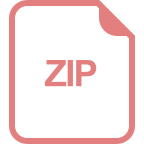
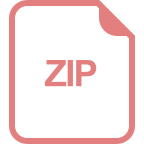
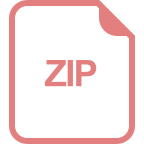














