用vue写一个弹出登录框组件
时间: 2023-06-09 22:04:49 浏览: 94
好的,下面是一个基于Vue的弹出登录框组件的代码示例:
```
<template>
<div class="login-modal">
<div class="modal">
<div class="modal-header">
<h4 class="modal-title">{{ title }}</h4>
<button type="button" class="close" @click="closeModal">×</button>
</div>
<div class="modal-body">
<form @submit.prevent="submitForm">
<div class="form-group">
<label for="username">用户名:</label>
<input type="text" class="form-control" v-model="username" />
</div>
<div class="form-group">
<label for="password">密码:</label>
<input type="password" class="form-control" v-model="password" />
</div>
<button type="submit" class="btn btn-primary">登录</button>
</form>
</div>
</div>
</div>
</template>
<script>
export default {
name: "LoginModal",
props: {
title: {
type: String,
default: "登录",
},
},
data() {
return {
username: "",
password: "",
};
},
methods: {
closeModal() {
this.$emit("close");
},
submitForm() {
// 发送登录请求
// ...
// 登录成功后关闭弹窗
this.closeModal();
},
},
};
</script>
<style>
.login-modal {
position: fixed;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: rgba(0, 0, 0, 0.5);
z-index: 9999;
display: flex;
justify-content: center;
align-items: center;
}
.modal {
max-width: 400px;
background-color: #fff;
border-radius: 5px;
padding: 20px;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.5);
}
.modal-header {
display: flex;
justify-content: space-between;
align-items: center;
}
.modal-title {
margin: 0;
font-size: 20px;
}
.close {
border: none;
background-color: transparent;
font-size: 24px;
cursor: pointer;
}
.form-group {
margin-bottom: 10px;
}
.form-control {
width: 100%;
padding: 8px;
border-radius: 5px;
border: 1px solid #ccc;
}
.btn-primary {
background-color: #007bff;
border: none;
color: #fff;
padding: 8px 16px;
border-radius: 5px;
cursor: pointer;
}
</style>
```
这个组件包含一个弹出框和一个表单,用户可以在表单中输入用户名和密码,点击登录按钮后发送登录请求。当登录成功后,弹出框会关闭。这个组件可以作为一个单独的模块来使用,只需要在父组件中调用并监听其`close`事件即可。
相关推荐
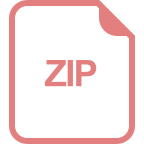













