使用java写一段nio的通信代码
时间: 2024-05-15 14:19:50 浏览: 25
以下是一个简单的 NIO 通信代码示例,其中使用了 Java 的 SocketChannel 和 Selector 类:
```java
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.SocketChannel;
import java.util.Iterator;
public class NIOClient {
private static final int BUFFER_SIZE = 1024;
private static final String HOST = "localhost";
private static final int PORT = 8080;
public static void main(String[] args) {
try {
SocketChannel socketChannel = SocketChannel.open();
socketChannel.configureBlocking(false);
Selector selector = Selector.open();
socketChannel.register(selector, SelectionKey.OP_CONNECT);
socketChannel.connect(new InetSocketAddress(HOST, PORT));
while (true) {
if (selector.select() <= 0) {
continue;
}
Iterator<SelectionKey> iterator = selector.selectedKeys().iterator();
while (iterator.hasNext()) {
SelectionKey key = iterator.next();
iterator.remove();
if (key.isConnectable()) {
SocketChannel channel = (SocketChannel) key.channel();
if (channel.isConnectionPending()) {
channel.finishConnect();
}
channel.configureBlocking(false);
channel.register(selector, SelectionKey.OP_WRITE);
System.out.println("Connected to server: " + HOST + ":" + PORT);
} else if (key.isWritable()) {
SocketChannel channel = (SocketChannel) key.channel();
ByteBuffer buffer = ByteBuffer.allocate(BUFFER_SIZE);
buffer.put("Hello, Server!".getBytes());
buffer.flip();
channel.write(buffer);
channel.register(selector, SelectionKey.OP_READ);
} else if (key.isReadable()) {
SocketChannel channel = (SocketChannel) key.channel();
ByteBuffer buffer = ByteBuffer.allocate(BUFFER_SIZE);
channel.read(buffer);
String message = new String(buffer.array()).trim();
System.out.println("Received message from server: " + message);
channel.close();
selector.close();
System.exit(0);
}
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
该示例使用了 Java 的 NIO 技术,通过 SocketChannel 和 Selector 类实现客户端与服务器之间的通信。在客户端中,首先创建一个 SocketChannel 对象,并将其配置为非阻塞模式。然后创建一个 Selector 对象并将 SocketChannel 注册到 Selector 上,设置监听事件为 OP_CONNECT。接着调用 connect() 方法来连接服务器。
在 while 循环中,首先使用 select() 方法阻塞等待事件发生。如果没有事件发生,则继续循环等待。如果有事件发生,则获取事件的 SelectionKey 并根据其对应的操作类型进行处理。如果是 OP_CONNECT 事件,则完成连接操作并将 SocketChannel 注册为 OP_WRITE 事件。如果是 OP_WRITE 事件,则向服务器发送数据,并将 SocketChannel 注册为 OP_READ 事件。如果是 OP_READ 事件,则从服务器读取数据,并关闭连接和 Selector。
注意,在这个示例中,我们只是发送了一条简单的字符串消息给服务器,并从服务器接收了一条回复消息。在实际应用中,您可能需要更复杂的协议和数据格式来进行通信。
相关推荐
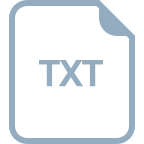
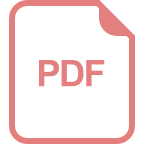
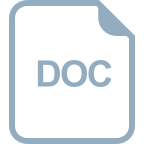
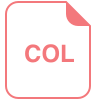
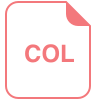
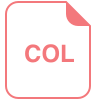
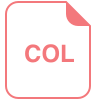
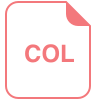










