tab切换实验内容vue
时间: 2023-07-09 13:05:41 浏览: 190
tab切换是一个常见的前端交互效果,可以通过Vue实现。以下是一个简单的实验,展示如何使用Vue实现tab切换效果:
1. 新建一个Vue组件,命名为Tab.vue,并在组件中定义tab数据和当前选中的tab索引:
```javascript
<template>
<div>
<div class="tab-header">
<div v-for="(tab, index) in tabs" :key="index" class="tab-item" :class="{ 'active': index === activeIndex }" @click="activeIndex = index">{{ tab.title }}</div>
</div>
<div class="tab-content">
<slot :name="tabs[activeIndex].name"></slot>
</div>
</div>
</template>
<script>
export default {
name: 'Tab',
props: {
tabs: {
type: Array,
required: true
}
},
data() {
return {
activeIndex: 0
}
}
}
</script>
<style scoped>
.tab-header {
display: flex;
}
.tab-item {
padding: 10px;
border: 1px solid #ccc;
cursor: pointer;
}
.tab-item.active {
background-color: #ccc;
}
.tab-content {
margin-top: 10px;
}
</style>
```
2. 在父组件中使用Tab组件,并传递tab数据:
```javascript
<template>
<div>
<tab :tabs="tabs">
<template v-for="(tab, index) in tabs" :key="index" :slot="tab.name">
{{ tab.content }}
</template>
</tab>
</div>
</template>
<script>
import Tab from './Tab.vue'
export default {
name: 'App',
components: {
Tab
},
data() {
return {
tabs: [
{ title: 'Tab 1', name: 'tab1', content: 'This is tab 1 content' },
{ title: 'Tab 2', name: 'tab2', content: 'This is tab 2 content' },
{ title: 'Tab 3', name: 'tab3', content: 'This is tab 3 content' }
]
}
}
}
</script>
```
3. 通过点击tab头部切换展示的内容:
```javascript
<template>
<div>
<div class="tab-header">
<div v-for="(tab, index) in tabs" :key="index" class="tab-item" :class="{ 'active': index === activeIndex }" @click="activeIndex = index">{{ tab.title }}</div>
</div>
<div class="tab-content">
<slot :name="tabs[activeIndex].name"></slot>
</div>
</div>
</template>
<script>
export default {
name: 'Tab',
props: {
tabs: {
type: Array,
required: true
}
},
data() {
return {
activeIndex: 0
}
}
}
</script>
<style scoped>
.tab-header {
display: flex;
}
.tab-item {
padding: 10px;
border: 1px solid #ccc;
cursor: pointer;
}
.tab-item.active {
background-color: #ccc;
}
.tab-content {
margin-top: 10px;
}
</style>
```
这样,就可以使用Vue实现tab切换效果了。
阅读全文
相关推荐
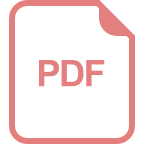
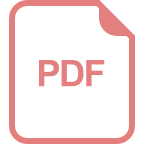
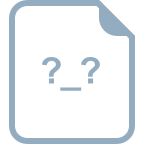
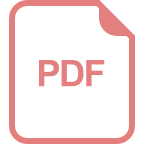
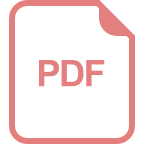
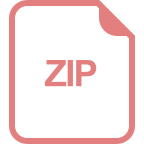
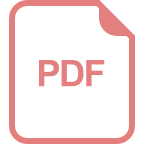
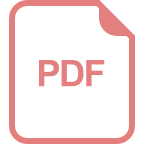
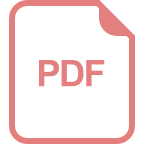
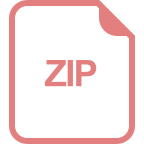
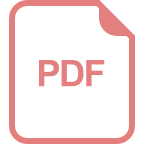
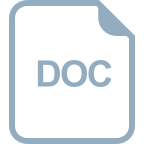