c++字符串中find
时间: 2024-09-18 14:05:35 浏览: 18
在C++中,`std::string` 类型提供了一个名为 `find()` 的成员函数,用于在一个字符串中查找另一个子串首次出现的位置。这个函数的原型如下:
```cpp
size_t find(const char* str, size_t pos = 0) const;
```
或者
```cpp
size_t find(std::string_view str, size_t pos = 0) const;
```
参数说明:
- `str`: 需要在原字符串中查找的目标子串,可以是一个字符指针 (`const char*`) 或者一个 `std::string_view` 对象。
- `pos` (可选): 查找的起始位置,默认从字符串的开头开始查找。
如果找到目标子串,`find()` 函数会返回该子串的第一个字符在原字符串中的索引(从0开始计数),如果是整数类型的 `pos`,则从那个位置开始搜索。如果没有找到,则返回 `npos`(通常表示 `std::string::npos`,即 `size_t(-1)`)。
例如:
```cpp
std::string str = "Hello, World!";
size_t index = str.find("World"); // 返回6,因为"World"从第7个字符开始
```
相关问题
c++字符串find函数
C语言中的字符串查找函数为strstr,而不是find函数。此函数用于在一个字符串中查找另一个指定的字符串,并返回第一次出现的位置,如果没有找到则返回NULL。函数原型为: char *strstr(const char *str1, const char *str2);
c++ 字符串find函数
C++中的字符串类(std::string)提供了find()函数,可以用于查找一个子串在字符串中出现的位置。它的语法如下:
```cpp
size_t find(const string& str, size_t pos = 0) const;
size_t find(const char* s, size_t pos = 0) const;
size_t find(const char* s, size_t pos, size_t n) const;
size_t find(char c, size_t pos = 0) const;
```
其中,第一个参数是要查找的子串,第二个参数是起始查找位置,默认为0。
示例:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "hello world";
int pos = str.find("world");
if (pos != string::npos) {
cout << "子串 \"world\" 在字符串 \"" << str << "\" 中的位置为:" << pos << endl;
} else {
cout << "子串 \"world\" 未找到!" << endl;
}
return 0;
}
```
输出结果:
```
子串 "world" 在字符串 "hello world" 中的位置为:6
```
相关推荐
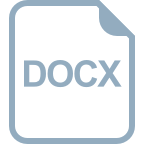
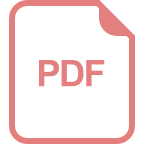
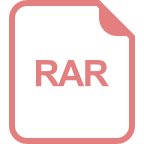

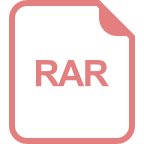
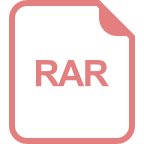
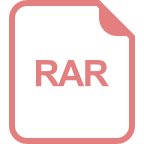
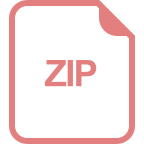
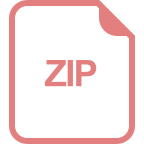
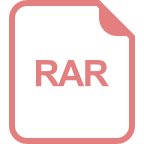
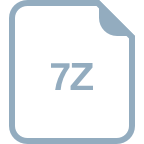
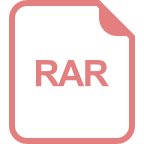
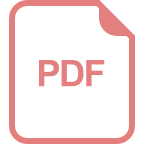


