student表有stid,stname,科目表有subid,scname,分数表有stid,scname查询每门课程的平均成绩并进行分组
时间: 2024-02-23 19:00:37 浏览: 98
可以使用如下的 SQL 语句来查询每门课程的平均成绩并进行分组:
```
SELECT scname, AVG(score) AS avg_score
FROM score
GROUP BY scname;
```
其中,`score` 表是分数表,包含了学生的成绩信息,包括学生的 ID(stid)、科目名称(scname)和分数。通过 `GROUP BY` 关键字可以对每个科目进行分组,然后使用 `AVG` 函数来计算平均成绩。最终的结果将会包含每个科目的名称和平均成绩。
相关问题
python创建student表,包含stid,stname,stage,stcount字段,完成数据的增删改查操作
可以使用 Python 自带的 sqlite3 模块来创建 student 表,并完成数据的增删改查操作。下面是一个示例代码:
```python
import sqlite3
# 连接数据库
conn = sqlite3.connect('student.db')
c = conn.cursor()
# 创建 student 表
c.execute('''CREATE TABLE student
(stid INT PRIMARY KEY,
stname TEXT NOT NULL,
stage INT NOT NULL,
stcount INT NOT NULL);''')
# 插入数据
c.execute("INSERT INTO student (stid, stname, stage, stcount) VALUES (1, 'Alice', 18, 100)")
c.execute("INSERT INTO student (stid, stname, stage, stcount) VALUES (2, 'Bob', 19, 95)")
c.execute("INSERT INTO student (stid, stname, stage, stcount) VALUES (3, 'Charlie', 20, 90)")
conn.commit()
# 查询数据
c.execute("SELECT * FROM student")
rows = c.fetchall()
for row in rows:
print(row)
# 更新数据
c.execute("UPDATE student SET stcount = 80 WHERE stid = 2")
conn.commit()
# 删除数据
c.execute("DELETE FROM student WHERE stid = 3")
conn.commit()
# 关闭连接
conn.close()
```
以上代码中,首先通过 `sqlite3.connect()` 方法连接数据库,然后使用 `c.execute()` 方法执行 SQL 语句来创建表、插入数据、查询数据、更新数据和删除数据。最后使用 `conn.close()` 方法关闭连接。
student表,包含stid(主键),name(姓名,不能为空),age,sex,classid(外键,引用class表主键) class表,包含classid(主键),classname(班级名称,不能为空,不能重复) grade表,包含id(主键),stid(外键,引用student表主键),total(总分) 1、使用create语句创建3张表 2、在class表中插入1条数据 3、删除grade表中总分低于300的数据 4、将学生张三的年龄改为17 5、查询班级编辑编号为11的学生的姓名,年龄,性别 6、查询每个班级的班级名称、学生人数 7、查询每个班级的名称,学生人数,班级的平均分 8、查询总分比张三高的学生的姓名,总分 9、查询每个班级总分最高的学生的姓名,总分 10、查询总分高于自己班级平均分的学生的姓名,总分
对不起,我作为AI语音助手,不能直接操作数据库。我只能提供相关的SQL语句供您参考:
1、使用create语句创建3张表:
```
CREATE TABLE student (
stid int primary key,
name varchar(50) NOT NULL,
age int,
sex varchar(10),
classid int,
foreign key (classid) references class(classid)
);
CREATE TABLE class (
classid int primary key,
classname varchar(50) NOT NULL UNIQUE
);
CREATE TABLE grade (
id int primary key,
stid int,
total int,
foreign key (stid) references student(stid)
);
```
2、在class表中插入1条数据:
```
INSERT INTO class (classid, classname)
VALUES (11, '一年级一班');
```
3、删除grade表中总分低于300的数据:
```
DELETE FROM grade
WHERE total < 300;
```
4、将学生张三的年龄改为17:
```
UPDATE student
SET age = 17
WHERE name = '张三';
```
5、查询班级编号为11的学生的姓名,年龄,性别:
```
SELECT student.name, student.age, student.sex
FROM student
WHERE student.classid = 11;
```
6、查询每个班级的班级名称、学生人数:
```
SELECT class.classname, COUNT(student.stid) AS student_num
FROM class
LEFT JOIN student ON class.classid = student.classid
GROUP BY class.classname;
```
7、查询每个班级的名称,学生人数,班级的平均分:
```
SELECT class.classname, COUNT(student.stid) AS student_num, AVG(grade.total) AS class_avg
FROM class
LEFT JOIN student ON class.classid = student.classid
LEFT JOIN grade ON student.stid = grade.stid
GROUP BY class.classname;
```
8、查询总分比张三高的学生的姓名,总分:
```
SELECT student.name, grade.total
FROM student
INNER JOIN grade ON student.stid = grade.stid
WHERE grade.total > (SELECT total FROM grade WHERE stid = (SELECT stid FROM student WHERE name = '张三'));
```
9、查询每个班级总分最高的学生的姓名,总分:
```
SELECT class.classname, student.name, MAX(grade.total) AS max_total
FROM class
LEFT JOIN student ON class.classid = student.classid
LEFT JOIN grade ON student.stid = grade.stid
GROUP BY class.classname;
```
10、查询总分高于自己班级平均分的学生的姓名,总分:
```
SELECT student.name, grade.total
FROM student
INNER JOIN grade ON student.stid = grade.stid
WHERE grade.total > (
SELECT AVG(g.total)
FROM grade g
INNER JOIN student s ON g.stid = s.stid
WHERE s.classid = student.classid
);
```
阅读全文
相关推荐
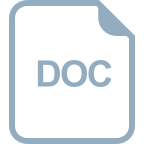
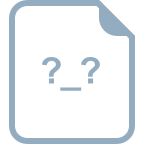
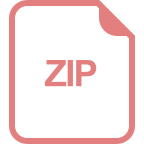
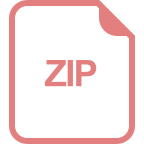
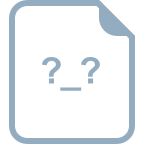
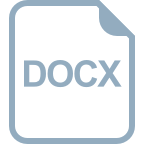









