std::string转rapidjson::Value
时间: 2024-10-17 11:01:02 浏览: 22
在RapidJSON库中,`std::string`通常用于表示JSON字符串,而`rapidjson::Value`则是处理JSON数据的基本结构。如果你有一个`std::string`类型的JSON字符串,想要转换成`rapidjson::Value`以便解析或操作,你可以按照以下步骤操作:
1. 首先,需要包含必要的头文件:
```cpp
#include "rapidjson/document.h"
```
2. 使用` rapidjson::Document::Parse `函数将字符串转换为` rapidjson::Value`对象。假设你的`std::string`叫做`jsonStr`:
```cpp
rapidjson::Document d;
if (d.Parse(jsonStr.c_str()).HasError()) {
// 处理解析错误
std::cout << "Failed to parse JSON." << std::endl;
} else {
// 成功解析,可以访问值了
}
```
这里,`HasError()`检查解析是否成功,如果失败则会返回一个错误状态。
相关问题
std::string转化成JSON::value
在C++中,将`std::string`转换为JSON库(如nlohmann/json或RapidJSON)中的`JSON::value`对象通常涉及到序列化字符串的过程。这里假设你使用的是nlohmann/json库,其步骤如下:
1. 首先,你需要包含必要的头文件:
```cpp
#include <nlohmann/json.hpp>
```
2. 使用`json::parse()`函数将字符串解析为`JSON::value`,这个函数尝试从给定的文本中解析JSON数据:
```cpp
std::string jsonString = "your_string_here";
nlohmann::json jsonValue = nlohmann::json::parse(jsonString);
```
如果字符串不是有效的JSON,这会抛出异常。
3. 现在`jsonValue`是一个JSON对象或数组,你可以像操作普通C++对象一样操作它了。
例如,如果你有一个简单的键值对字符串 `"name": "John"`:
```cpp
std::string jsonString = "{\"name\": \"John\"}";
nlohmann::json jsonValue = nlohmann::json::parse(jsonString);
const std::string name = jsonValue["name"].get<std::string>(); // 获取名字
```
rapidjson::document.addmember写入std::string
在RapidJSON中,如果你想要向`Document`对象添加成员,其中成员值是`std::string`类型,你可以这样做:
首先,确保你已经包含了必要的头文件:
```cpp
#include <rapidjson/document.h>
#include <rapidjson/writer.h>
#include <fstream>
#include <string>
```
假设你有一个`Document`对象`d`,并想添加一个新的键值对,键是`std::string`类型的`key`,值是`std::string`类型的`value`:
```cpp
// 初始化文档对象
rapidjson::Document d;
// 创建一个std::string类型的键
std::string key = "yourKey";
// 创建一个std::string类型的值
std::string value = "yourValue"; // 这里替换为你实际的字符串
// 使用addMember方法添加成员
d.AddMember(key.c_str(), value.c_str(), d.GetAllocator()); // 使用const char*指针传递字符串
// 你也可以选择使用PrettyWriter增加可读性,但在这里为了简洁只列出基本的AddMember
// rapidjson::Document d;
// rapidjson::PrettyWriter<rapidjson::StringBuffer> writer(d.GetAllocator());
// writer.AddMember(key, value, d.GetAllocator());
// 然后,你可以像之前提到的那样,将整个Document序列化到文件或字符串流中。
```
这里的`GetAllocator()`是用来确保内存管理的一致性的,因为RapidJSON会自动管理内存,所以需要提供一个合适的内存分配器。
阅读全文
相关推荐
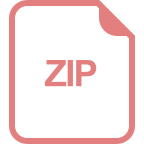
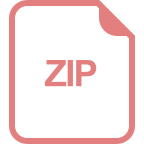
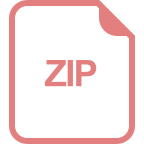






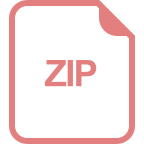
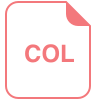





