代码实现hashmap
时间: 2023-09-02 13:05:39 浏览: 37
可以给您讲解一下哈希表的原理以及如何实现一个简单的HashMap。
哈希表是一种通过将键映射到索引来访问数据的数据结构。在哈希表中,每个键值对被映射到一个唯一的索引,这个索引通常是使用哈希函数计算出来的。
实现一个简单的HashMap,首先需要定义一个数组用来存储键值对。接下来,要实现一个哈希函数,这个哈希函数需要将键转换成一个唯一的整数,然后用这个整数对数组的大小取模,得到一个索引。
当需要插入一个键值对时,首先将键用哈希函数进行转换得到索引,然后将键值对存储到对应的索引位置。当需要查找一个键值对时,同样使用哈希函数进行转换得到索引,然后在对应的索引位置查找键值对是否存在。
以下是一个简单的HashMap的实现例子:
```java
public class HashMap {
private static final int SIZE = 16;
private Entry[] entries = new Entry[SIZE];
public void put(String key, Object value) {
int index = getHashCode(key) % SIZE;
Entry entry = entries[index];
if (entry == null) {
entries[index] = new Entry(key, value);
} else {
while (entry.next != null && !entry.key.equals(key)) {
entry = entry.next;
}
if (entry.key.equals(key)) {
entry.value = value;
} else {
entry.next = new Entry(key, value);
}
}
}
public Object get(String key) {
int index = getHashCode(key) % SIZE;
Entry entry = entries[index];
while (entry != null && !entry.key.equals(key)) {
entry = entry.next;
}
return entry == null ? null : entry.value;
}
private int getHashCode(String key) {
int hash = 0;
for (char c : key.toCharArray()) {
hash = 31 * hash + c;
}
return hash;
}
private static class Entry {
private final String key;
private Object value;
private Entry next;
public Entry(String key, Object value) {
this.key = key;
this.value = value;
next = null;
}
}
}
```
以上是一个基于链表的哈希表实现,当哈希值相同时,将键值对存储在链表中。实际生产环境中需要考虑哈希冲突,还需要实现哈希表的扩容等功能。
相关推荐
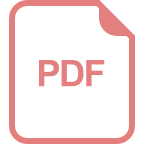














