c++实现 hashmap
时间: 2024-02-27 21:50:28 浏览: 24
C++中可以使用标准库中的unordered_map来实现哈希表(hashmap)。unordered_map是一个关联容器,它使用哈希函数将键映射到值,从而实现快速的查找。
以下是一个简单的示例代码,展示了如何使用unordered_map实现哈希表:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
// 创建一个unordered_map对象
std::unordered_map<int, std::string> hashmap;
// 向哈希表中插入键值对
hashmap.insert({1, "apple"});
hashmap.insert({2, "banana"});
hashmap.insert({3, "orange"});
// 查找键为2的值
std::cout << "Value at key 2: " << hashmap[2] << std::endl;
// 遍历哈希表
for (const auto& pair : hashmap) {
std::cout << "Key: " << pair.first << ", Value: " << pair.second << std::endl;
}
return 0;
}
```
运行上述代码,输出结果为:
```
Value at key 2: banana
Key: 1, Value: apple
Key: 2, Value: banana
Key: 3, Value: orange
```
在上述示例中,我们首先创建了一个unordered_map对象,然后使用insert函数向哈希表中插入键值对。通过使用键来访问值,我们可以使用类似于数组的语法`hashmap[key]`来获取对应的值。最后,我们使用范围for循环遍历整个哈希表,并输出每个键值对的内容。
相关推荐
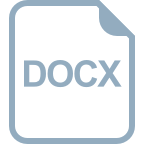














