c++ hashmap 例子
时间: 2023-07-23 19:04:59 浏览: 109
下面是一个使用C++标准库中的`std::unordered_map`实现的哈希表的例子:
```c++
#include <iostream>
#include <unordered_map>
#include <string>
int main() {
// 创建一个哈希表
std::unordered_map<std::string, int> hashMap;
// 添加键值对
hashMap["apple"] = 5;
hashMap["banana"] = 2;
hashMap["orange"] = 8;
// 访问元素
std::cout << "The value of apple is: " << hashMap["apple"] << std::endl;
// 修改元素
hashMap["banana"] = 10;
// 检查键是否存在
if (hashMap.count("orange") > 0) {
std::cout << "The key 'orange' exists in the hashmap." << std::endl;
}
// 遍历哈希表
for (const auto& pair : hashMap) {
std::cout << "Key: " << pair.first << ", Value: " << pair.second << std::endl;
}
return 0;
}
```
在上面的示例中,我们首先创建了一个`std::unordered_map<std::string, int>`类型的哈希表,键的类型是`std::string`,值的类型是`int`。然后,我们通过使用`[]`操作符添加键值对,并使用`count`方法检查键是否存在。我们还可以使用迭代器遍历哈希表中的键值对。最后,我们使用`std::cout`打印了一些信息。
请注意,`std::unordered_map`是一个无序哈希表,它提供了O(1)的平均插入、查找和删除操作的性能。
阅读全文
相关推荐



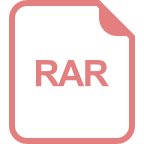
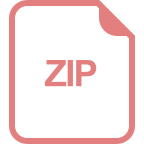
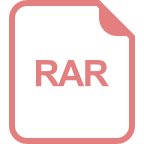
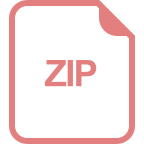
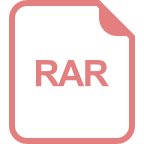
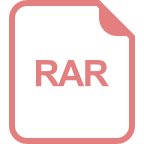
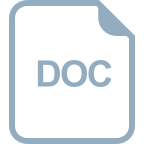
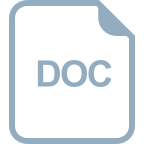
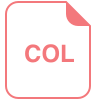
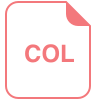
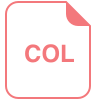
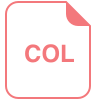
