webots python A*算法 路径规划算法
时间: 2023-09-24 13:14:29 浏览: 207
Webots是一个流行的机器人仿真平台,它提供了Python API来让用户可以使用Python编写控制机器人的代码。A*算法是一种经典的路径规划算法,可以在网格地图上寻找最短路径。
下面是一个使用A*算法进行路径规划的Python代码示例,假设机器人在(0,0)位置,目标点为(5,5),地图大小为10x10。
```python
import heapq
# 定义地图大小
MAP_SIZE = 10
# 定义障碍物
obstacles = [(2, 2), (2, 3), (2, 4), (3, 4), (4, 4), (5, 4), (6, 4), (6, 3), (6, 2)]
# 定义起点和终点
start = (0, 0)
end = (5, 5)
# 定义节点类
class Node:
def __init__(self, x, y, g=0, h=0, parent=None):
self.x = x
self.y = y
self.g = g
self.h = h
self.f = g + h
self.parent = parent
def __lt__(self, other):
return self.f < other.f
def __eq__(self, other):
return self.x == other.x and self.y == other.y
# 定义A*算法函数
def A_star(start, end):
open_list = []
closed_list = []
heapq.heappush(open_list, Node(start[0], start[1]))
while open_list:
current_node = heapq.heappop(open_list)
if current_node == Node(end[0], end[1]):
path = []
while current_node:
path.append((current_node.x, current_node.y))
current_node = current_node.parent
return path[::-1]
closed_list.append(current_node)
for dx, dy in [(0, 1), (0, -1), (1, 0), (-1, 0)]:
neighbor_node = Node(current_node.x + dx, current_node.y + dy, current_node.g + 1,
abs(current_node.x + dx - end[0]) + abs(current_node.y + dy - end[1]), current_node)
if neighbor_node.x < 0 or neighbor_node.x >= MAP_SIZE or neighbor_node.y < 0 or neighbor_node.y >= MAP_SIZE:
continue
if neighbor_node in obstacles:
continue
if neighbor_node in closed_list:
continue
if neighbor_node not in open_list:
heapq.heappush(open_list, neighbor_node)
return None
# 测试
path = A_star(start, end)
print(path)
```
该代码会输出一个从起点到终点的路径,如果路径不存在则输出None。
阅读全文
相关推荐





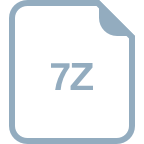
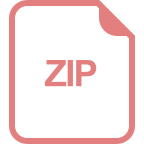
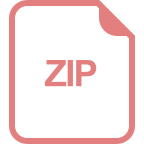
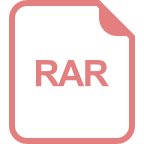
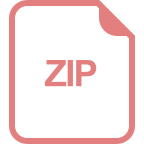
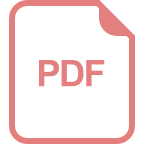
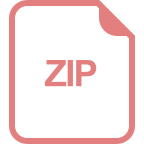
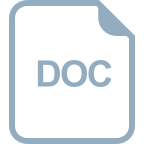
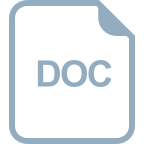
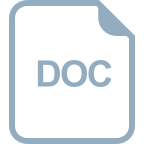
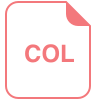