编写运用GDAL并且可以在visual stdio中实现高斯滤波的c++的代码
时间: 2024-10-19 15:05:18 浏览: 59
在C++中使用GDAL(Geospatial Data Abstraction Library)进行高斯滤波通常涉及地理空间数据处理库和图像操作。以下是一个简单的示例,展示了如何在Visual Studio中使用GDAL对栅格数据进行高斯滤波。请注意,这只是一个基本框架,并未包含完整的项目设置和错误处理:
```cpp
#include "gdal_priv.h"
#include <iostream>
// 高斯滤波函数
void ApplyGaussianFilter(const char* inputRaster, const char* outputRaster, double sigma) {
GDALDataset* dataset = (GDALDataset*) GDALOpen(inputRaster, GA_ReadOnly);
if (!dataset) {
std::cerr << "Error opening raster file: " << inputRaster << std::endl;
return;
}
// 获取栅格带宽
int width = dataset->RasterXSize;
int height = dataset->RasterYSize;
// 创建输出文件
GDALDriver* driver = GetGDALDriverManager()->GetDriverByName("GTiff");
if (!driver) {
std::cerr << "Error: Could not find GTiff driver." << std::endl;
return;
}
GDALDataset* outputFile = driver->Create(outputRaster, width, height, 1, GDT_Float32, nullptr);
if (!outputFile) {
std::cerr << "Error creating output file." << std::endl;
return;
}
// 获取输入数据Band
GDALRasterBand* bandIn = dataset->GetRasterBand(1);
GDALRasterBand* bandOut = outputFile->GetRasterBand(1);
// 应用高斯滤波
double noDataValue = bandIn->GetNoDataValue();
GDALRasterMathOperation op = GAUSSIAN;
double* outbuf = static_cast<double*>(bandOut->GetRasterBlock()->GetRawRaster());
double* inbuf = static_cast<double*>(bandIn->RasterIO(GF_Read, 0, 0, width, height, nullptr, width, height, GDT_Float32));
for (int y = 0; y < height; ++y) {
for (int x = 0; x < width; ++x) {
// 这里省略了计算高斯权重的部分,你需要实际实现这部分
float filteredValue = CalculateFilteredValue(inbuf[x], sigma, noDataValue); // 假设有一个CalculateFilteredValue函数
if (noDataValue != -9999 && noDataValue == inbuf[x]) { // 如果是无效值,则保持不变
outbuf[y * width + x] = noDataValue;
} else {
outbuf[y * width + x] = filteredValue;
}
}
}
// 清理内存并保存
delete[] inbuf;
bandOut->RasterIO(GF_Write, 0, 0, width, height, outbuf, width, height, GDT_Float32);
bandIn->RasterIO(GF_Read, 0, 0, width, height, nullptr, width, height, GDT_Float32);
GDALClose(dataset);
outputFile->FlushCache();
// 关闭文件
delete outputFile;
}
// 用于简化演示,这里假设有一个CalculateFilteredValue函数
double CalculateFilteredValue(double value, double sigma, double noDataValue) {
// 实现高斯滤波公式,例如OpenCV中的filter2D
// 省略具体数学计算,因为此处仅作展示
return value;
}
// 主函数
int main() {
// 输入和输出文件路径
const char* inputRaster = "input.tif";
const char* outputRaster = "filtered.tif";
// 设置高斯滤波标准差
double sigma = 2.0;
ApplyGaussianFilter(inputRaster, outputRaster, sigma);
return 0;
}
```
阅读全文
相关推荐
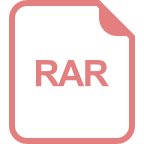
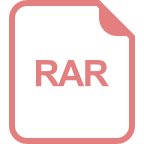
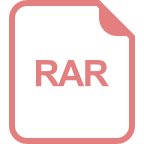



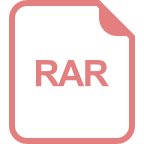
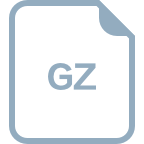
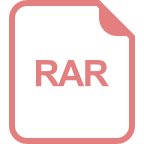
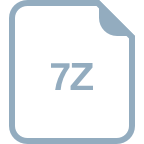
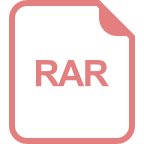
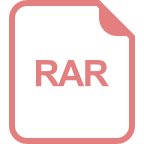
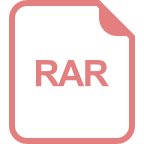




