package com.songhaozhi.mayday.service; import java.util.List; import com.songhaozhi.mayday.model.domain.Category; /** * @author : 宋浩志 * @createDate : 2018年9月26日 * */ public interface CategoryService { /** * 查询所有分类 * * @return */ List<Category> findCategory(); /** * 根据id查询 * * @param categoryId * @return */ Category findByCategoryId(int categoryId); /** * 添加 * * @param category */ void save(Category category) throws Exception; /** * 修改 * * @param category */ void update(Category category) throws Exception; /** * 删除 * * @param categoryId */ void delete(int categoryId) throws Exception; /** * 验证是否存在该路径 * * @param cateUrl * @return */ Category findByCateUrl(String cateUrl); } 编写测试用例,测试ArticleService的insert、findAllArticle和countByStatus方法。
时间: 2023-07-23 20:07:17 浏览: 55
下面是针对`ArticleService`的三个方法编写的测试用例示例:
```java
import org.junit.Assert;
import org.junit.Before;
import org.junit.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import java.util.ArrayList;
import java.util.List;
public class ArticleServiceTest {
@Mock
private ArticleRepository articleRepository;
@InjectMocks
private ArticleService articleService;
@Before
public void setup() {
MockitoAnnotations.initMocks(this);
}
@Test
public void testInsert() {
// 创建模拟数据
Article article = new Article();
// 设置模拟行为
when(articleRepository.insert(article)).thenReturn(true);
// 调用被测试方法
boolean result = articleService.insert(article);
// 断言预期结果与实际结果是否一致
Assert.assertTrue(result);
}
@Test
public void testFindAllArticle() {
// 创建模拟数据
List<Article> expectedArticles = new ArrayList<>();
expectedArticles.add(new Article());
expectedArticles.add(new Article());
// 设置模拟行为
when(articleRepository.findAllArticle()).thenReturn(expectedArticles);
// 调用被测试方法
List<Article> actualArticles = articleService.findAllArticle();
// 断言预期结果与实际结果是否一致
Assert.assertEquals(expectedArticles, actualArticles);
}
@Test
public void testCountByStatus() {
// 创建模拟数据
int status = 1;
int expectedCount = 5;
// 设置模拟行为
when(articleRepository.countByStatus(status)).thenReturn(expectedCount);
// 调用被测试方法
int actualCount = articleService.countByStatus(status);
// 断言预期结果与实际结果是否一致
Assert.assertEquals(expectedCount, actualCount);
}
}
```
在这些示例中,我们使用了`@Mock`注解来模拟`ArticleRepository`依赖,并使用`@InjectMocks`注解将`ArticleService`类注入到测试类中。然后,我们使用`when`方法设置模拟行为,并调用被测试方法进行测试,最后使用断言来验证预期结果与实际结果是否一致。
希望以上示例能够帮助你编写测试用例。如果你还有其他问题,请随时提问。
相关推荐
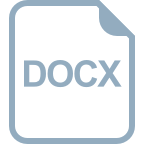
















