题目描述:你需要设计一个控制台地图游戏,玩家需要通过控制台输入来移动自己的角色,并在地图上寻找宝藏。玩家可以通过键盘控制小球向上、向下、向左或向右移动。当小球移动到宝藏处时,游戏结束,输出恭喜信息。 要求: 1. 可以通过输入行数和列数调整地图的大小; 2. 可以随机生成宝藏位置和角色起始位置,注意两者不能相同,借助rand()函数生成; 提示:可以采用w、s、a、d表示键盘控制的方向。游戏开始时用O表示角色起始位置,X表示宝藏所在位置。当到达目的地后,即X和O符号重合时变成“$”符号。 示例: 请输入地图的行数和列数:8 8 ########## #O.......# #.x......# #........# #........# #........# #........# #........# #........# ########## 请输入移动方向(w/a/s/d): s ########## #........# #Ox......# #........# #........# #........# #........# #........# #........# ########## 请输入移动方向(w/a/s/d): d ########## #........# #.$......# #........# #........# #........# #........# #........# #........# ########## 游戏结束,恭喜你完成游戏。
时间: 2023-12-06 10:44:31 浏览: 71
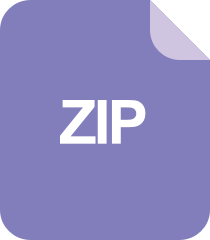
基于C++控制台的吃豆人小游戏【100012333】

以下是一个可能的实现,包含注释以方便理解:
```cpp
#include <iostream>
#include <cstdlib> // 包含rand()和srand()函数
using namespace std;
const char PLAYER_SYMBOL = 'O';
const char TREASURE_SYMBOL = 'X';
const char FINISH_SYMBOL = '$';
int main() {
// 设置随机数种子,以当前时间作为种子
srand(time(NULL));
int rows, cols;
cout << "请输入地图的行数和列数:";
cin >> rows >> cols;
// 创建地图
char map[rows][cols];
// 随机生成角色和宝藏的位置
int playerRow = rand() % rows;
int playerCol = rand() % cols;
int treasureRow, treasureCol;
do {
treasureRow = rand() % rows;
treasureCol = rand() % cols;
} while (treasureRow == playerRow && treasureCol == playerCol);
// 初始化地图
for (int row = 0; row < rows; row++) {
for (int col = 0; col < cols; col++) {
if (row == playerRow && col == playerCol) {
map[row][col] = PLAYER_SYMBOL;
} else if (row == treasureRow && col == treasureCol) {
map[row][col] = TREASURE_SYMBOL;
} else {
map[row][col] = '#';
}
}
}
// 游戏循环
bool gameOver = false;
while (!gameOver) {
// 输出地图
for (int row = 0; row < rows; row++) {
for (int col = 0; col < cols; col++) {
cout << map[row][col];
}
cout << endl;
}
// 获取玩家输入的移动方向
char direction;
cout << "请输入移动方向(w/a/s/d):";
cin >> direction;
// 根据移动方向更新角色位置
int newPlayerRow = playerRow, newPlayerCol = playerCol;
switch (direction) {
case 'w':
newPlayerRow--;
break;
case 's':
newPlayerRow++;
break;
case 'a':
newPlayerCol--;
break;
case 'd':
newPlayerCol++;
break;
}
// 判断新位置是否合法,如果合法则更新角色位置
if (newPlayerRow >= 0 && newPlayerRow < rows && newPlayerCol >= 0 && newPlayerCol < cols) {
// 如果新位置是宝藏所在位置,游戏结束
if (newPlayerRow == treasureRow && newPlayerCol == treasureCol) {
map[playerRow][playerCol] = FINISH_SYMBOL;
gameOver = true;
} else {
map[playerRow][playerCol] = '.'; // 把旧位置变成空地
map[newPlayerRow][newPlayerCol] = PLAYER_SYMBOL;
playerRow = newPlayerRow;
playerCol = newPlayerCol;
}
}
}
// 输出恭喜信息
cout << "游戏结束,恭喜你完成游戏。" << endl;
return 0;
}
```
阅读全文
相关推荐
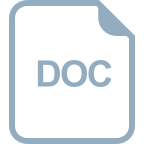
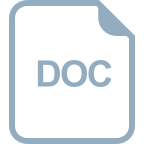





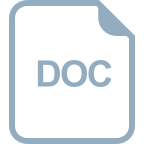
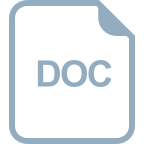
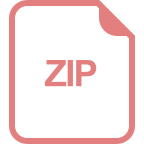
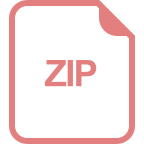
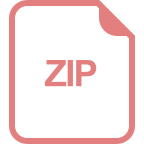