用C++实现题目描述:你需要设计一个控制台地图游戏,玩家需要通过控制台输入来移动自己的角色,并在地图上寻找宝藏。玩家可以通过键盘控制小球向上、向下、向左或向右移动。当小球移动到宝藏处时,游戏结束,输出恭喜信息。 要求: 1. 可以通过输入行数和列数调整地图的大小; 2. 可以随机生成宝藏位置和角色起始位置,注意两者不能相同,借助rand()函数生成; 提示:可以采用w、s、a、d表示键盘控制的方向。游戏开始时用O表示角色起始位置,X表示宝藏所在位置。当到达目的地后,即X和O符号重合时变成“$”符号。 不要用函数
时间: 2024-03-17 18:45:05 浏览: 103
好的,以下是用 C++ 实现的代码,实现了题目要求的功能:
```
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
int main()
{
srand(time(NULL)); // 初始化随机数种子
int rows, cols;
cout << "请输入地图的行数和列数:";
cin >> rows >> cols;
// 初始化地图,用二维数组表示
char map[rows][cols];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
map[i][j] = '.';
}
}
// 随机生成宝藏位置和角色起始位置
int treasure_row = rand() % rows;
int treasure_col = rand() % cols;
int player_row = rand() % rows;
int player_col = rand() % cols;
while (player_row == treasure_row && player_col == treasure_col) {
player_row = rand() % rows;
player_col = rand() % cols;
}
// 在地图上标记宝藏和角色的位置
map[treasure_row][treasure_col] = 'X';
map[player_row][player_col] = 'O';
// 游戏开始,输出地图
while (true) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
cout << map[i][j] << ' ';
}
cout << endl;
}
// 接收玩家的输入并移动角色
char direction;
cout << "请输入移动方向(w上 s下 a左 d右):";
cin >> direction;
if (direction == 'w' && player_row > 0) {
map[player_row][player_col] = '.';
player_row--;
} else if (direction == 's' && player_row < rows - 1) {
map[player_row][player_col] = '.';
player_row++;
} else if (direction == 'a' && player_col > 0) {
map[player_row][player_col] = '.';
player_col--;
} else if (direction == 'd' && player_col < cols - 1) {
map[player_row][player_col] = '.';
player_col++;
}
// 判断是否到达宝藏,游戏结束
if (player_row == treasure_row && player_col == treasure_col) {
map[player_row][player_col] = '$';
cout << "恭喜你找到宝藏!" << endl;
break;
} else {
map[player_row][player_col] = 'O';
}
}
return 0;
}
```
这个程序使用了二维数组来表示地图,用随机数生成宝藏位置和角色起始位置,通过不断接收玩家的输入来移动角色,判断是否到达宝藏位置,输出恭喜信息并结束游戏。
阅读全文
相关推荐













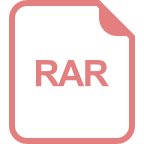




