如何对mnist数据添加高斯噪声
时间: 2023-07-02 12:20:07 浏览: 186
要对 MNIST 数据集添加高斯噪声,您可以使用 Python 的 NumPy 和 OpenCV 库。下面是一些基本步骤:
1. 从 MNIST 数据集中加载图像数据
2. 将图像数据转换为 NumPy 数组
3. 使用 OpenCV 的高斯模糊函数添加高斯噪声
4. 再次将 NumPy 数组转换为图像数据
以下是一个示例代码,可以为您提供更好的理解:
```python
import cv2
import numpy as np
from tensorflow.keras.datasets import mnist
# 加载 MNIST 数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 将图像数据转换为 NumPy 数组
x_train = np.array(x_train)
x_test = np.array(x_test)
# 添加高斯噪声
sigma = 50 # 噪声强度
x_train_noisy = np.zeros(x_train.shape)
x_test_noisy = np.zeros(x_test.shape)
for i in range(x_train.shape[0]):
noise = np.random.normal(0, sigma, x_train[i].shape)
x_train_noisy[i] = cv2.add(x_train[i], noise, dtype=cv2.CV_64F)
for i in range(x_test.shape[0]):
noise = np.random.normal(0, sigma, x_test[i].shape)
x_test_noisy[i] = cv2.add(x_test[i], noise, dtype=cv2.CV_64F)
# 将 NumPy 数组转换为图像数据
x_train_noisy = x_train_noisy.reshape(x_train_noisy.shape[0], 28, 28, 1)
x_test_noisy = x_test_noisy.reshape(x_test_noisy.shape[0], 28, 28, 1)
```
在这个示例中,我们使用了 `cv2.add()` 函数来将高斯噪声添加到图像中。`sigma` 参数可以控制噪声的强度。最后,我们再次使用 `reshape()` 函数将 NumPy 数组转换回图像数据。
相关推荐
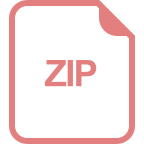
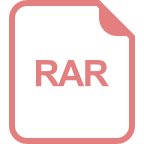
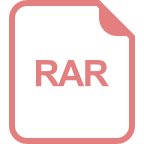














