使用Mybatis-Plus查询5条数据
时间: 2023-04-07 20:03:37 浏览: 72
可以回答这个问题。使用Mybatis-Plus查询5条数据的代码如下:
```
List<User> userList = userService.list(new QueryWrapper<User>().last("limit 5"));
```
其中,User是实体类,userService是对应的Service类。这段代码使用了Mybatis-Plus提供的QueryWrapper类,通过last方法设置limit限制查询结果数量为5条。
相关问题
mybatis-plus查询一条数据
使用Mybatis-Plus查询一条数据可以通过以下步骤实现:
1. 导入Mybatis-Plus的依赖包。
2. 创建一个实体类,用于映射数据库中的表。
3. 创建一个Mapper接口,继承BaseMapper接口,并指定泛型为实体类。
4. 在Mapper接口中定义一个查询方法,使用@Select注解,并指定SQL语句。
5. 在Service层中调用Mapper接口的查询方法,获取查询结果。
6. 在Controller层中将查询结果返回给前端。
示例代码如下:
1. 导入依赖包
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.3.1</version>
</dependency>
```
2. 创建实体类
```java
@Data
public class User {
private Long id;
private String name;
private Integer age;
private String email;
}
```
3. 创建Mapper接口
```java
public interface UserMapper extends BaseMapper<User> {
@Select("SELECT * FROM user WHERE id = #{id}")
User selectById(Long id);
}
```
4. 在Service层中调用Mapper接口的查询方法
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public User getUserById(Long id) {
return userMapper.selectById(id);
}
}
```
5. 在Controller层中将查询结果返回给前端
```java
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id);
}
}
```
以上就是使用Mybatis-Plus查询一条数据的步骤。
使用Mybatis-Plus查询倒数5条数据
可以使用Mybatis-Plus提供的Wrapper类来实现查询倒数5条数据的功能。具体实现如下:
```
QueryWrapper wrapper = new QueryWrapper();
wrapper.orderByDesc("id").last("limit 5");
List<Entity> entityList = entityMapper.selectList(wrapper);
```
其中,"id"为实体类中的主键字段名,"Entity"为实体类名,"entityMapper"为Mybatis-Plus自动生成的Mapper接口。使用orderByDesc方法可以按照id降序排列,使用last方法可以在SQL语句末尾添加"limit 5",限制查询结果为倒数5条数据。最后使用selectList方法执行查询并返回结果。
相关推荐
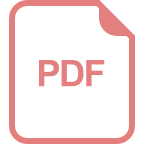
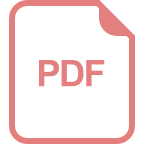
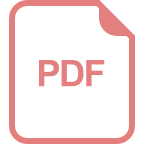












