基于python的使用orb特征图像的图像拼接代码
时间: 2024-02-27 14:52:36 浏览: 105
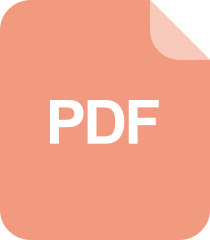
Python实现图片拼接的代码
下面是一个基于Python使用ORB特征图像进行图像拼接的示例代码:
```python
import cv2
import numpy as np
# 读取图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 将图像转换为灰度图像
gray1 = cv2.cvtColor(img1, cv2.COLOR_BGR2GRAY)
gray2 = cv2.cvtColor(img2, cv2.COLOR_BGR2GRAY)
# 创建ORB对象
orb = cv2.ORB_create()
# 检测特征点和描述符
kp1, des1 = orb.detectAndCompute(gray1, None)
kp2, des2 = orb.detectAndCompute(gray2, None)
# 匹配特征点
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
matches = bf.match(des1, des2)
# 将匹配结果按照距离进行排序
matches = sorted(matches, key=lambda x: x.distance)
# 取前10个匹配结果
good_matches = matches[:10]
# 获取匹配点对的坐标
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
# 寻找变换矩阵
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 将图像1变换到与图像2相同的坐标系下
h, w = gray1.shape
pts = np.float32([[0, 0], [0, h - 1], [w - 1, h - 1], [w - 1, 0]]).reshape(-1, 1, 2)
dst = cv2.perspectiveTransform(pts, M)
# 计算图像拼接后的大小
x_min = int(min(dst[:, :, 0])[0])
y_min = int(min(dst[:, :, 1])[0])
x_max = int(max(dst[:, :, 0])[0])
y_max = int(max(dst[:, :, 1])[0])
width = x_max - x_min
height = y_max - y_min
# 在新的画布上拼接图像
result = np.zeros((height, width, 3), np.uint8)
result[-y_min:height-y_min, -x_min:width-x_min] = img1
result = cv2.warpPerspective(result, M, (width, height))
# 显示拼接结果
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上述代码中,我们首先读取了两张图像,并将它们转换为灰度图像。然后,我们创建了ORB对象,使用detectAndCompute方法检测特征点和描述符。接着,我们使用BFMatcher算法进行特征点匹配,并根据距离对匹配结果进行排序,取前10个匹配结果。然后,我们获取匹配点对的坐标,使用findHomography方法寻找变换矩阵,将图像1变换到与图像2相同的坐标系下。最后,我们计算图像拼接后的大小,在新的画布上拼接图像,并显示拼接结果。
阅读全文
相关推荐
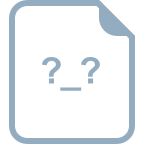


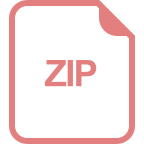
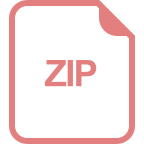
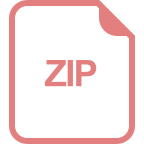
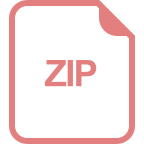
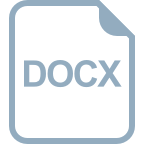
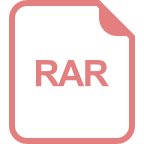
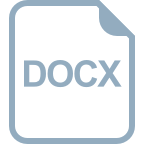
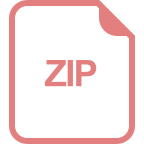
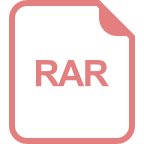
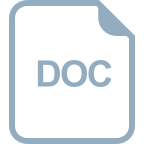



