qt网络编程简易计算器代码
时间: 2023-11-12 15:59:35 浏览: 100
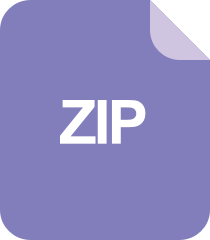
qt计算器简单代码
好的,这是一个简单的Qt网络编程计算器的代码示例:
```cpp
#include <QtWidgets>
#include <QtNetwork>
class Calculator : public QWidget
{
Q_OBJECT
public:
Calculator(QWidget *parent = nullptr);
private slots:
void requestNewConnection();
void readClientData();
void sendResult();
private:
QTcpServer *tcpServer;
QTcpSocket *clientSocket;
QTextEdit *display;
quint16 blockSize;
QString currentOperation;
double currentResult;
};
Calculator::Calculator(QWidget *parent)
: QWidget(parent), tcpServer(new QTcpServer(this)), clientSocket(nullptr),
display(new QTextEdit(this)), blockSize(0), currentOperation(""), currentResult(0.0)
{
if (!tcpServer->listen(QHostAddress::Any, 1234)) {
QMessageBox::critical(this, tr("Error"), tr("Unable to start the server: %1.").arg(tcpServer->errorString()));
close();
return;
}
connect(tcpServer, &QTcpServer::newConnection, this, &Calculator::requestNewConnection);
QVBoxLayout *layout = new QVBoxLayout(this);
layout->addWidget(display);
}
void Calculator::requestNewConnection()
{
if (clientSocket) {
clientSocket->disconnectFromHost();
clientSocket->deleteLater();
}
clientSocket = tcpServer->nextPendingConnection();
connect(clientSocket, &QTcpSocket::readyRead, this, &Calculator::readClientData);
}
void Calculator::readClientData()
{
QDataStream in(clientSocket);
in.setVersion(QDataStream::Qt_5_15);
if (blockSize == 0) {
if (clientSocket->bytesAvailable() < static_cast<int>(sizeof(quint16)))
return;
in >> blockSize;
}
if (clientSocket->bytesAvailable() < blockSize)
return;
QString operation;
in >> operation;
if (operation == "clear") {
currentResult = 0.0;
currentOperation = "";
display->setText("");
} else if (operation == "+" || operation == "-" || operation == "*" || operation == "/") {
currentOperation = operation;
currentResult = display->toPlainText().toDouble();
display->setText("");
} else if (operation == "=") {
double operand = display->toPlainText().toDouble();
if (currentOperation == "+")
currentResult += operand;
else if (currentOperation == "-")
currentResult -= operand;
else if (currentOperation == "*")
currentResult *= operand;
else if (currentOperation == "/")
currentResult /= operand;
display->setText(QString::number(currentResult));
}
sendResult();
}
void Calculator::sendResult()
{
QByteArray block;
QDataStream out(&block, QIODevice::WriteOnly);
out.setVersion(QDataStream::Qt_5_15);
out << static_cast<quint16>(0);
out << display->toPlainText();
out.device()->seek(0);
out << static_cast<quint16>(block.size() - sizeof(quint16));
clientSocket->write(block);
blockSize = 0;
}
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Calculator calculator;
calculator.show();
return app.exec();
}
```
这个计算器使用TCP协议进行通信,监听端口号为1234。当客户端连接到服务器时,服务器会接收客户端发送的数据,并根据数据进行计算,然后将计算结果发送回客户端。
阅读全文
相关推荐
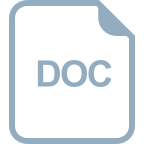
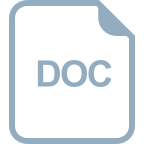
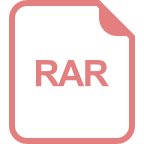
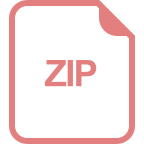
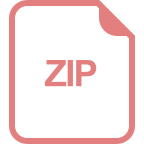
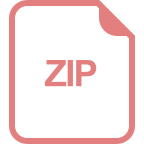
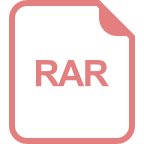
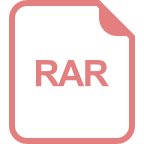
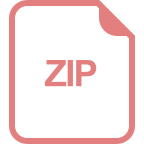
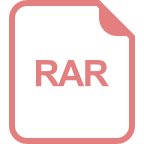
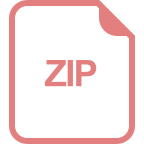
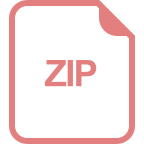
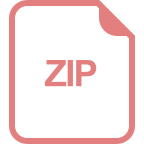
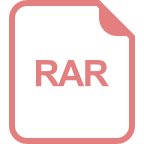
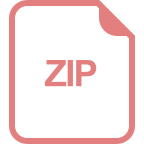
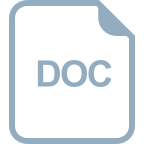