MyBatis@Select三表联合查询后,可以将查询结果赋给实体类吗
时间: 2023-08-03 21:04:53 浏览: 123
可以的,MyBatis@Select三表联合查询后,可以将查询结果赋给实体类。在Mapper.xml文件中,我们可以使用join语句来进行多表联合查询,然后在@Select注解中指定SQL语句,查询结果会自动映射到实体类中。需要注意的是,实体类的属性名称必须与查询结果集中的列名一致,否则需要使用别名来进行映射。同时,我们还需要在实体类上使用@Result注解来指定每个属性对应的列名,以及进行类型转换等操作。例如:
```java
public class User {
private Integer id;
private String name;
private Integer age;
// 省略getter和setter方法
}
@ResultType(User.class)
@Select("SELECT u.id, u.name, u.age FROM user u JOIN order o ON u.id = o.user_id JOIN order_item oi ON o.id = oi.order_id WHERE oi.product_id = #{productId}")
List<User> selectUsersByProductId(Integer productId);
```
在上面的例子中,我们使用了@ResultType注解来指定查询结果映射到User类中,然后使用了@Select注解指定了SQL语句,最终查询结果会自动映射到User类中。
相关问题
mybatis-plus三表查询
MyBatis-Plus的三表查询可以通过使用MyBatis的XML映射文件或者注解方式来实现。以下是一个使用XML映射文件实现的三表查询示例:
1. 创建实体类
假设我们有三个实体类:User、Role和UserRole,分别对应数据库中的三张表。
User实体类:
```java
public class User {
private Long id;
private String username;
private String password;
private String email;
// getter和setter方法
}
```
Role实体类:
```java
public class Role {
private Long id;
private String roleName;
private String description;
// getter和setter方法
}
```
UserRole实体类:
```java
public class UserRole {
private Long id;
private Long userId;
private Long roleId;
// getter和setter方法
}
```
2. 创建Mapper接口
创建三个Mapper接口:UserMapper、RoleMapper和UserRoleMapper,分别对应User、Role和UserRole实体类。
UserMapper接口:
```java
public interface UserMapper extends BaseMapper<User> {}
```
RoleMapper接口:
```java
public interface RoleMapper extends BaseMapper<Role> {}
```
UserRoleMapper接口:
```java
public interface UserRoleMapper extends BaseMapper<UserRole> {}
```
3. 创建XML映射文件
在MyBatis的XML映射文件中定义三个SQL语句,分别查询用户、角色和用户角色关联信息。
UserMapper.xml文件:
```xml
<mapper namespace="com.example.mapper.UserMapper">
<resultMap id="userRoleMap" type="com.example.entity.User">
<id column="id" property="id" />
<result column="username" property="username" />
<result column="password" property="password" />
<result column="email" property="email" />
<collection property="roles" ofType="com.example.entity.Role">
<id column="role_id" property="id" />
<result column="role_name" property="roleName" />
<result column="description" property="description" />
</collection>
</resultMap>
<select id="getUserWithRolesById" resultMap="userRoleMap">
select u.id, u.username, u.password, u.email, r.id as role_id, r.role_name, r.description
from user u
left join user_role ur on u.id = ur.user_id
left join role r on ur.role_id = r.id
where u.id = #{id}
</select>
</mapper>
```
RoleMapper.xml文件:
```xml
<mapper namespace="com.example.mapper.RoleMapper">
<select id="getRoleWithUsersById" resultType="com.example.entity.Role">
select r.id, r.role_name, r.description, u.id as user_id, u.username, u.password, u.email
from role r
left join user_role ur on r.id = ur.role_id
left join user u on ur.user_id = u.id
where r.id = #{id}
</select>
</mapper>
```
UserRoleMapper.xml文件:
```xml
<mapper namespace="com.example.mapper.UserRoleMapper">
<select id="getUserRoleByUserId" resultType="com.example.entity.UserRole">
select id, user_id, role_id from user_role where user_id = #{userId}
</select>
</mapper>
```
4. 使用三表查询
在Service层或Controller层中调用Mapper接口中定义的方法即可使用三表查询功能。
UserService类:
```java
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
@Autowired
private UserRoleMapper userRoleMapper;
@Autowired
private RoleMapper roleMapper;
public User getUserWithRolesById(Long id) {
User user = userMapper.getUserWithRolesById(id);
List<UserRole> userRoles = userRoleMapper.getUserRoleByUserId(user.getId());
for (UserRole userRole : userRoles) {
Role role = roleMapper.getRoleWithUsersById(userRole.getRoleId());
user.getRoles().add(role);
}
return user;
}
}
```
RoleService类:
```java
@Service
public class RoleService {
@Autowired
private RoleMapper roleMapper;
@Autowired
private UserRoleMapper userRoleMapper;
@Autowired
private UserMapper userMapper;
public Role getRoleWithUsersById(Long id) {
Role role = roleMapper.getRoleWithUsersById(id);
List<UserRole> userRoles = userRoleMapper.getUserRoleByRoleId(role.getId());
for (UserRole userRole : userRoles) {
User user = userMapper.getUserWithRolesById(userRole.getUserId());
role.getUsers().add(user);
}
return role;
}
}
```
注:以上示例中的XML映射文件中的SQL语句仅供参考,实际使用时需要根据业务需求自行修改。
mybatis实体类与表映射
MyBatis 是一款持久层框架,可以通过 XML 文件或注解形式进行配置,将 Java 实体类与数据库表进行映射。下面是一个示例:
1. 实体类定义
```java
public class User {
private Long id;
private String username;
private String password;
private Integer age;
// getter 和 setter 略
}
```
2. 数据库表定义
```sql
CREATE TABLE user (
id BIGINT(20) NOT NULL AUTO_INCREMENT,
username VARCHAR(50) DEFAULT NULL,
password VARCHAR(50) DEFAULT NULL,
age INT(11) DEFAULT NULL,
PRIMARY KEY (id)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
3. MyBatis XML 文件配置
```xml
<!-- UserMapper.xml -->
<mapper namespace="com.example.mapper.UserMapper">
<resultMap id="userMap" type="com.example.entity.User">
<id column="id" property="id" />
<result column="username" property="username" />
<result column="password" property="password" />
<result column="age" property="age" />
</resultMap>
<select id="getUserById" resultMap="userMap">
SELECT id, username, password, age FROM user WHERE id = #{id}
</select>
<insert id="insertUser" parameterType="com.example.entity.User">
INSERT INTO user (username, password, age) VALUES (#{username}, #{password}, #{age})
</insert>
<update id="updateUser" parameterType="com.example.entity.User">
UPDATE user SET username = #{username}, password = #{password}, age = #{age} WHERE id = #{id}
</update>
<delete id="deleteUserById">
DELETE FROM user WHERE id = #{id}
</delete>
</mapper>
```
4. Mapper 接口定义
```java
public interface UserMapper {
User getUserById(Long id);
void insertUser(User user);
void updateUser(User user);
void deleteUserById(Long id);
}
```
这样就完成了 Java 实体类与数据库表的映射。在实际开发中,可以根据具体需求进行配置,比如使用注解方式配置映射关系,或者使用 MyBatis-Plus 等工具简化操作。
阅读全文
相关推荐
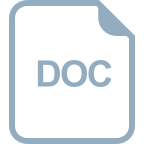
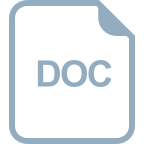
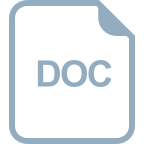
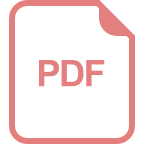
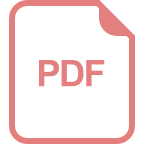
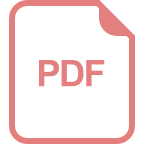
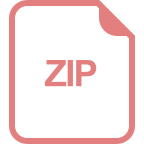
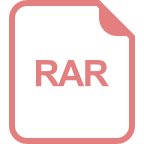
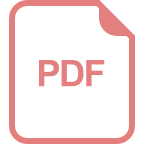







