根据行数列数左上角右上角右下角算出矩阵所有坐标及间距,要求C Shap语言封装
时间: 2023-05-31 08:03:54 浏览: 88
以下是一个C#语言的矩阵类,其中包含计算矩阵所有坐标及间距的方法:
```
public class Matrix
{
private int[,] data;
private int rows;
private int cols;
public Matrix(int rows, int cols)
{
this.rows = rows;
this.cols = cols;
this.data = new int[rows, cols];
}
public int Rows { get { return rows; } }
public int Cols { get { return cols; } }
public int this[int row, int col]
{
get { return data[row, col]; }
set { data[row, col] = value; }
}
public void FillRandom()
{
Random rand = new Random();
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
data[i, j] = rand.Next(100);
}
}
}
public void Print()
{
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
Console.Write("{0}\t", data[i, j]);
}
Console.WriteLine();
}
}
public List<Point> GetAllPoints()
{
List<Point> points = new List<Point>();
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
points.Add(new Point(i, j));
}
}
return points;
}
public double GetSpacing()
{
Point topLeft = new Point(0, 0);
Point topRight = new Point(0, cols - 1);
Point bottomRight = new Point(rows - 1, cols - 1);
double horizontalSpacing = (topRight.X - topLeft.X) / (double)(cols - 1);
double verticalSpacing = (bottomRight.Y - topRight.Y) / (double)(rows - 1);
return Math.Sqrt(horizontalSpacing * horizontalSpacing + verticalSpacing * verticalSpacing);
}
}
```
使用方法:
```
Matrix matrix = new Matrix(3, 4);
matrix.FillRandom();
matrix.Print();
List<Point> points = matrix.GetAllPoints();
foreach (Point p in points)
{
Console.WriteLine("({0}, {1})", p.X, p.Y);
}
double spacing = matrix.GetSpacing();
Console.WriteLine("Spacing: {0}", spacing);
```
阅读全文
相关推荐
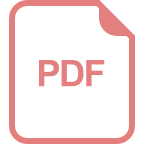
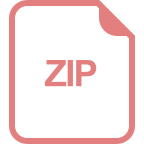
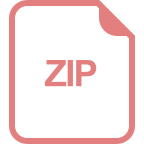


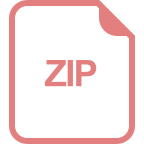
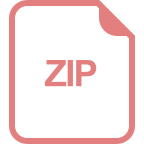
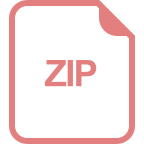
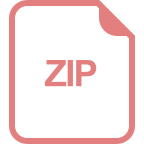
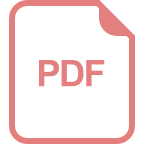
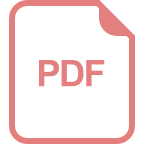
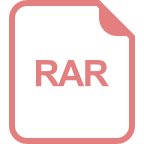
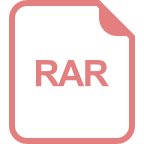
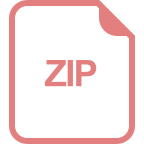
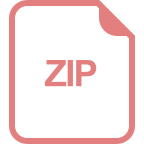
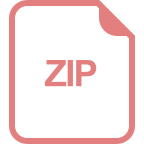