c语言用单链表实现两个大整数相加运算 目的:深入掌握单链表应用的算法设计 主要功能: 1、将用户输入的十进制整数字符串转化为带头结点的单链表,每个结点存放一个整数位 2、求两个整数单链表相加的结果单链表 3、求结果单链表的中间位,如123的中间位为2,1234的中间位为2
时间: 2024-10-17 17:11:41 浏览: 14
在C语言中,我们可以使用单链表来实现两个大整数相加操作。首先,我们需要创建一个链表节点结构体,包含一个整数值域和指向下一个节点的指针。下面是一个简化的步骤:
1. **创建链表节点**:
```c
typedef struct ListNode {
int value;
struct ListNode* next;
} ListNode;
```
2. **初始化链表头**:
```c
ListNode* createList(const char* str) {
ListNode* head = NULL, *current = NULL;
for (int i = 0; str[i]; ++i) {
if (str[i] == '\0') break; // 检查是否到达字符串结尾
ListNode newNode;
newNode.value = str[i] - '0'; // 转换字符到数字
newNode.next = head;
head = &newNode;
}
return head;
}
```
3. **合并两个链表并相加**:
```c
void addTwoLists(ListNode* list1, ListNode* list2, ListNode* result) {
ListNode* temp1 = list1, *temp2 = list2, *currResult = result;
int carry = 0;
while (list1 || list2) {
int sum = carry + (temp1 ? temp1->value : 0);
sum += (temp2 ? temp2->value : 0);
currResult->value = sum % 10;
carry = sum / 10;
if (temp1) temp1 = temp1->next;
if (temp2) temp2 = temp2->next;
currResult->next = (carry || temp1 || temp2) ? malloc(sizeof(ListNode)) : NULL;
currResult = currResult->next;
}
if (carry > 0) {
ListNode newNode;
newNode.value = carry;
newNode.next = NULL;
currResult->next = &newNode;
}
}
```
4. **打印链表**:
```c
void printList(ListNode* head) {
while (head) {
printf("%d", head->value);
head = head->next;
}
printf("\n");
}
// 主函数示例
int main() {
char str1[] = "123";
char str2[] = "456";
ListNode* list1 = createList(str1);
ListNode* list2 = createList(str2);
ListNode* resultHead = malloc(sizeof(ListNode));
resultHead->value = 0;
addTwoLists(list1, list2, resultHead);
printList(resultHead);
return 0;
}
```
阅读全文
相关推荐
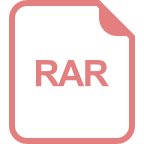
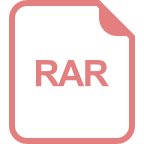
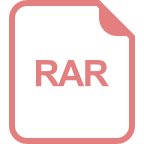

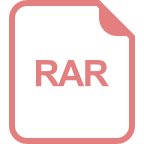
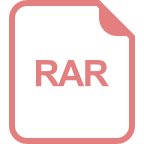
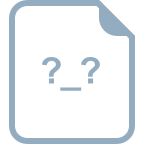
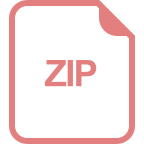
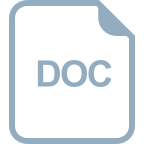
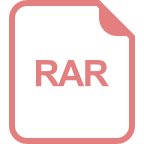
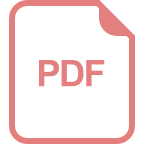
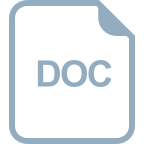
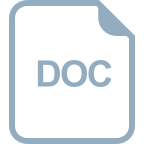
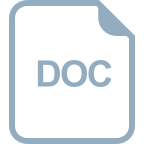
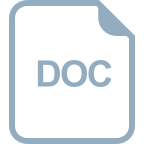
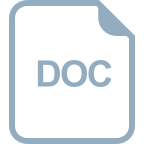
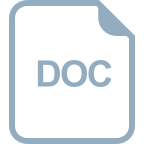
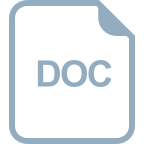
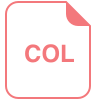