输出文件中单词的数量(将其中所有标点符号替换为空格,再根据空格对字符串进行切分可以得到元素为单词的列表。数字按单词统计,如“ 1994” 计算一个单词)
时间: 2024-10-18 18:06:35 浏览: 41
为了计算文件中单词的数量,你需要先读取文件内容,移除所有的标点符号并将连续的空格替换为单个空格。然后,你可以使用split()函数将文本分割成单词列表,最后统计列表的长度即为单词总数。对于包含数字的单词,可以使用正则表达式进一步处理。以下是一个简单的Python代码示例:
```python
import re
def count_words_in_file(file_path):
word_count = 0
with open(file_path, 'r') as file:
text = file.read().replace(',', ' ').replace('.', ' ').replace('!', ' ').replace(':', ' ')
words = re.findall(r'\b\w+\b', text) # 正则表达式匹配单词
word_count = len(words)
# 对于包含数字的单词,这里简单处理将其视为一个单独的词
word_count += sum(1 for word in words if word.isdigit())
return word_count
# 调用函数并打印结果
word_count = count_words_in_file('your_file.txt')
print(f"文件中有{word_count}个单词")
相关问题
python输出文件中单词的数量,将其中所有标点符号替换为空格,再根据空格对字符串进行切分可以得到元素为单词的列表。数字按单词统计
在Python中,你可以使用`re`模块进行正则表达式操作,先去除标点符号,然后读取文件内容,对每行文本进行处理并统计单词数量。这里是一个简单的步骤:
1. 导入必要的库:
```python
import re
```
2. 定义函数来处理文件内容:
```python
def count_words_in_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
# 使用正则表达式替换标点符号,并转换成小写
cleaned_content = re.sub(r'[^\w\s]', '', content).lower()
# 利用split()函数根据空格分割字符串,得到单词列表
words_list = cleaned_content.split()
# 统计单词频率
word_count = {word: words_list.count(word) for word in words_list}
return word_count
```
3. 调用函数并打印结果:
```python
file_path = "your_file.txt" # 替换为你需要分析的文件路径
word_counts = count_words_in_file(file_path)
for word, count in word_counts.items():
print(f"{word}: {count}")
在右侧编辑器中的 Begin-End 区间补充代码,统计单词数量。具体要求如下: 输出文件中单词的数量(将其中所有标点符号替换为空格,再根据空格对字符串进行切分可以得到元素为单词的列表。数字按单词统计,如 1994 计算一个单词)。
你可以使用Python编写一个简单的脚本来完成这个任务。这里是一个示例代码片段:
```python
import string
def count_words(text):
# 移除标点符号并将文本转换为小写
text = text.translate(str.maketrans('', '', string.punctuation)).lower()
# 分割单词并计算数量
words = text.split()
word_count = {word: words.count(word) for word in set(words)} # 使用集合去除重复,计数每个单词出现的次数
return word_count
# 假设右侧编辑器中的begin-end区间存储了文本内容
text_in_editor = editor_content[begin:end]
word_count = count_words(text_in_editor)
with open("output_file.txt", "w") as f:
for word, count in word_count.items():
f.write(f"{word}: {count}\n")
阅读全文
相关推荐
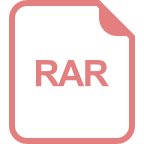
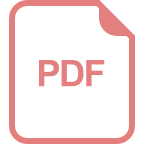
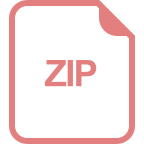










