public object UnityEnghine { get; private set; }在C#中什么意思
时间: 2024-04-19 17:27:28 浏览: 19
在C#中,这行代码定义了一个名为 UnityEnghine 的属性,其类型为 object。这个属性具有一个公共的 getter 方法和一个私有的 setter 方法。换句话说,外部代码可以读取 UnityEnghine 属性的值,但只有类内部的代码可以修改它的值。这种访问控制方式通常用于限制对属性的写入权限,以确保只有类内部的方法可以修改属性的值。
相关问题
C# 带get set的变量有什么区别
C# 中带有 get 和 set 方法的变量被称为属性(Property)。属性提供了一种简单和安全的方式,用于访问和修改对象的私有字段或计算的值。
区别如下:
1. 访问控制:属性可以帮助控制对变量的访问权限,因为可以在 get 和 set 方法中添加访问控制修饰符。例如,可以将 get 方法设置为 public 而将 set 方法设置为 private,这样只能获取属性值,而不能设置属性值。
2. 计算值:属性可以计算值并返回,因此可以在 get 方法中编写代码来计算属性的值,而不是简单地返回变量的值。
3. 数据校验:属性可以帮助校验数据的有效性,例如,在 set 方法中添加一些条件语句,以确保输入的值符合预期的条件。
4. 语法简洁:使用属性可以使代码更加简洁易懂,例如,可以使用类似于 myObject.MyProperty 的语法来访问属性,而不是使用 myObject.GetMyProperty() 和 myObject.SetMyProperty() 的方法。
c#实例方法是什么意思
C#中的实例方法是指属于类的对象的方法。它们需要通过创建类的实例来调用。实例方法可以访问和操作类的实例变量和属性,并且可以在方法内部使用this关键字来引用当前对象。
以下是一个示例,演示了如何定义和调用C#中的实例方法:
```csharp
using System;
class MyClass
{
private int myVariable;
public void SetVariable(int value)
{
myVariable = value;
}
public int GetVariable()
{
return myVariable;
}
}
class Program
{
static void Main(string[] args)
{
MyClass myObject = new MyClass(); // 创建一个类的实例
myObject.SetVariable(10); // 调用实例方法设置变量的值
int result = myObject.GetVariable(); // 调用实例方法获取变量的值
Console.WriteLine("Variable value: " + result); // 输出:Variable value: 10
}
}
```
在上面的示例中,我们定义了一个名为MyClass的类,其中包含了两个实例方法SetVariable和GetVariable。在Main方法中,我们创建了一个MyClass的实例myObject,并使用该实例调用了SetVariable和GetVariable方法来设置和获取变量的值。
相关推荐
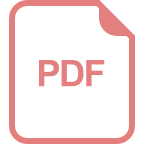
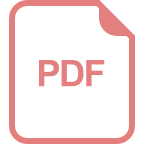
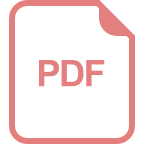












