masm十六进制转换为字符串
时间: 2024-11-13 17:14:49 浏览: 12
在MASM (Microsoft Assembly) 中,将十六进制数转换成字符串通常涉及以下步骤:
1. 定义存储区:首先,你需要一个缓冲区来存放转换后的字符串结果。这个缓冲区需要足够的空间来存储十六进制数字及其前导零(如果有的话)。
2. 初始化变量:设置一个循环计数器、一个用于当前位的临时变量(例如DX),以及指向缓冲区起始位置的指针。
3. 获取十六进制值:假设你想转换的十六进制数存放在内存某个位置,比如eax。你可以从eax开始,每次左移一位并获取最低四位作为十进制数值。
4. 转换:对于每一位,你可以使用`shr eax, 4` 和 `and eax, 0x0F` 来提取最低四位,然后通过查表或者其他方法将其转换为对应的字符(如'A'到'F')。如果是小于10的数字,则直接对应ASCII码即可。
5. 添加到字符串:将转换得到的字符写入缓冲区,并更新计数器和指针。如果遇到最高位(最高四位全为0),说明已经处理完所有的十六进制位。
6. 结束处理:当计数器等于十六进制数总位数时,停止循环,字符串处理完成。
下面是一个简化版的例子:
```assembly
section .data
hex_string db '0123456789ABCDEF',0
buffer_size equ 16
; 具体十六进制值在eax
start_address dd hex_string
current_index dd buffer_size - 1
section .text
global _start
_start:
push ebx ; save ebx for later use
mov ebx, eax ; copy the hex value to ebx for shifting
convert_hex_loop:
shr ebx, 4 ; shift right by 4 bits
and ebx, 0x0F ; keep only lowest four bits
add al, ebx ; add to ASCII character
cmp ebx, 0 ; check if we've shifted all bits
jnz convert_hex_loop ; if not, continue
mov byte [start_address + current_index], al ; store the char in buffer
inc current_index ; move to next position in buffer
loop convert_hex_loop ; repeat until done
; now add null terminator
mov byte [start_address + current_index], 0
; restore ebx and exit
pop ebx
ret
```
阅读全文
相关推荐
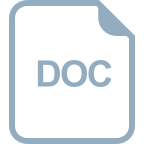
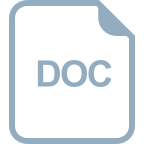
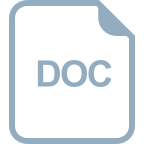
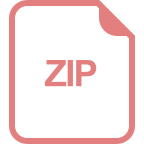



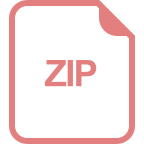
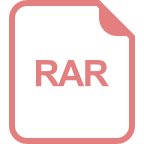
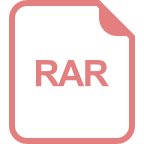
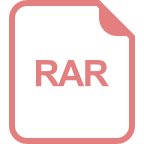
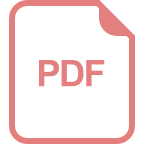
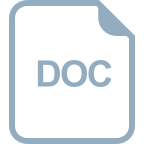
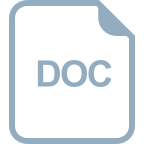



