定义一个结构体Student,包含学生的姓名、学号和成绩。请编写一个函数,输入一个学生数组和数组长度,输出成绩最高的学生的姓名和年龄。
时间: 2024-04-09 18:31:25 浏览: 27
下面是一个示例代码,实现了输入一个学生数组和数组长度,输出成绩最高的学生的姓名和成绩:
```c
#include <stdio.h>
#include <string.h>
#define MAX_NAME_LENGTH 50
// 定义学生结构体
struct Student {
char name[MAX_NAME_LENGTH];
int studentID;
float score;
};
// 函数声明:找出成绩最高的学生
void findHighestScoreStudent(struct Student* students, int length);
int main() {
int length;
printf("请输入学生数组的长度:");
scanf("%d", &length);
// 声明学生数组
struct Student students[length];
// 输入学生信息
for (int i = 0; i < length; i++) {
printf("请输入第%d个学生的姓名:", i+1);
scanf("%s", students[i].name);
printf("请输入第%d个学生的学号:", i+1);
scanf("%d", &students[i].studentID);
printf("请输入第%d个学生的成绩:", i+1);
scanf("%f", &students[i].score);
}
// 调用函数输出成绩最高的学生信息
findHighestScoreStudent(students, length);
return 0;
}
// 函数定义:找出成绩最高的学生
void findHighestScoreStudent(struct Student* students, int length) {
int maxIndex = 0;
// 遍历找出成绩最高的学生
for (int i = 1; i < length; i++) {
if (students[i].score > students[maxIndex].score) {
maxIndex = i;
}
}
// 输出成绩最高的学生信息
printf("成绩最高的学生姓名:%s\n", students[maxIndex].name);
printf("成绩最高的学生学号:%d\n", students[maxIndex].studentID);
printf("成绩最高的学生成绩:%.2f\n", students[maxIndex].score);
}
```
在上述代码中,我们首先定义了一个`Student`结构体,包含了学生的姓名(字符数组)、学号(整数)和成绩(浮点数)。然后,我们定义了`findHighestScoreStudent()`函数,它接收一个指向学生数组的指针和数组长度作为参数,用于找出成绩最高的学生。
在`main()`函数中,我们首先输入学生数组的长度,并声明了一个对应长度的学生数组。然后,通过循环输入每个学生的姓名、学号和成绩。最后,调用`findHighestScoreStudent()`函数输出成绩最高的学生的信息。
注意:在实际应用中,可以根据需要对输入进行错误处理和边界检查。此处仅作为示例代码,未进行详尽的输入验证。
相关推荐
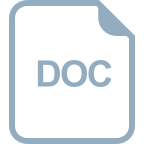
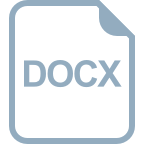
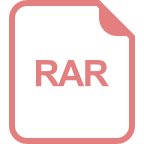














