用c语言实现学生信息管理系统中删除学生的功能,不能使用链表,初始化学生信息和删除学生信息都用函数完成
时间: 2024-03-22 07:39:36 浏览: 79
好的,这里给出一种使用函数完成初始化和删除学生信息的方法,具体实现步骤如下:
1. 定义一个结构体来存储学生信息,包括学号、姓名、性别、年龄等字段。
```c
struct Student {
int id;
char name[20];
char gender;
int age;
};
```
2. 定义一个数组来存储学生信息,可以使用静态数组或动态数组,这里以静态数组为例。
```c
#define MAX_STUDENTS 100
struct Student students[MAX_STUDENTS];
int count = 0; // 记录当前学生数量
```
3. 定义一个初始化函数来添加学生信息,使用指针作为参数,方便传递数组和数组长度。
```c
void initStudents(struct Student *students, int *count) {
students[0].id = 1001;
strcpy(students[0].name, "张三");
students[0].gender = 'M';
students[0].age = 20;
students[1].id = 1002;
strcpy(students[1].name, "李四");
students[1].gender = 'F';
students[1].age = 19;
*count = 2;
}
```
4. 定义一个删除学生的函数,使用指针作为参数,方便修改数组和数组长度。
```c
void deleteStudent(struct Student *students, int *count, int id) {
int i;
for (i = 0; i < *count; i++) {
if (students[i].id == id) {
break;
}
}
if (i == *count) {
printf("没有找到该学生!\n");
return;
}
for (int j = i; j < *count - 1; j++) {
students[j] = students[j + 1];
}
(*count)--;
printf("删除成功!\n");
}
```
完整代码如下:
```c
#include <stdio.h>
#define MAX_STUDENTS 100
struct Student {
int id;
char name[20];
char gender;
int age;
};
void initStudents(struct Student *students, int *count) {
students[0].id = 1001;
strcpy(students[0].name, "张三");
students[0].gender = 'M';
students[0].age = 20;
students[1].id = 1002;
strcpy(students[1].name, "李四");
students[1].gender = 'F';
students[1].age = 19;
*count = 2;
}
void deleteStudent(struct Student *students, int *count, int id) {
int i;
for (i = 0; i < *count; i++) {
if (students[i].id == id) {
break;
}
}
if (i == *count) {
printf("没有找到该学生!\n");
return;
}
for (int j = i; j < *count - 1; j++) {
students[j] = students[j + 1];
}
(*count)--;
printf("删除成功!\n");
}
int main() {
struct Student students[MAX_STUDENTS];
int count = 0;
// 初始化学生信息
initStudents(students, &count);
// 删除学号为1002的学生
deleteStudent(students, &count, 1002);
// 输出剩余学生信息
for (int i = 0; i < count; i++) {
printf("学号:%d,姓名:%s,性别:%c,年龄:%d\n", students[i].id, students[i].name, students[i].gender, students[i].age);
}
return 0;
}
```
希望能够帮到您!
阅读全文
相关推荐
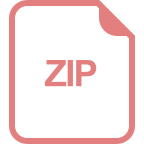
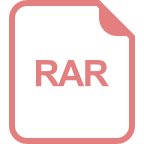
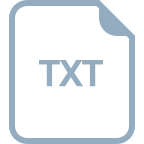















